md5加密算法实现c++
时间: 2023-09-15 15:23:02 浏览: 127
好的,关于md5加密算法的c实现,我可以为您提供以下代码:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <math.h>
#include <stdint.h>
#define LEFTROTATE(x, c) (((x) << (c)) | ((x) >> (32 - (c))))
void md5(uint8_t *initial_msg, size_t initial_len, uint8_t *digest) {
// These vars will contain the hash
uint32_t h0, h1, h2, h3;
// Message (to prepare)
uint8_t *msg = NULL;
// Note: All variables are unsigned 32 bit and wrap modulo 2^32 when calculating
// r specifies the per-round shift amounts
uint32_t r[] = {
7, 12, 17, 22,
7, 12, 17, 22,
7, 12, 17, 22,
7, 12, 17, 22,
5, 9, 14, 20,
5, 9, 14, 20,
5, 9, 14, 20,
5, 9, 14, 20,
4, 11, 16, 23,
4, 11, 16, 23,
4, 11, 16, 23,
4, 11, 16, 23,
6, 10, 15, 21,
6, 10, 15, 21,
6, 10, 15, 21,
6, 10, 15, 21
};
// Use binary integer part of the sines of integers (Radians) as constants:
const uint32_t k[] = {
0xd76aa478, 0xe8c7b756, 0x242070db, 0xc1bdceee,
0xf57c0faf, 0x4787c62a, 0xa8304613, 0xfd469501,
0x698098d8, 0x8b44f7af, 0xffff5bb1, 0x895cd7be,
0x6b901122, 0xfd987193, 0xa679438e, 0x49b40821,
0xf61e2562, 0xc040b340, 0x265e5a51, 0xe9b6c7aa,
0xd62f105d, 0x2441453, 0xd8a1e681, 0xe7d3fbc8,
0x21e1cde6, 0xc33707d6, 0xf4d50d87, 0x455a14ed,
0xa9e3e905, 0xfcefa3f8, 0x676f02d9, 0x8d2a4c8a,
0xfffa3942, 0x8771f681, 0x6d9d6122, 0xfde5380c,
0xa4beea44, 0x4bdecfa9, 0xf6bb4b60, 0xbebfbc70,
0x289b7ec6, 0xeaa127fa, 0xd4ef3085, 0x4881d05,
0xd9d4d039, 0xe6db99e5, 0x1fa27cf8, 0xc4ac5665,
0xf4292244, 0x432aff97, 0xab9423a7, 0xfc93a039,
0x655b59c3, 0x8f0ccc92, 0xffeff47d, 0x85845dd1,
0x6fa87e4f, 0xfe2ce6e0, 0xa3014314, 0x4e0811a1,
0xf7537e82, 0xbd3af235, 0x2ad7d2bb, 0xeb86d391
};
// Initialize variables:
h0 = 0x67452301;
h1 = 0xEFCDAB89;
h2 = 0x98BADCFE;
h3 = 0x10325476;
// Pre-processing:
// append "1" bit to message
// append "0" bits until message length in bits ≡ 448 (mod 512)
// append length mod (2^64) to message
// (Prepended to message)
// append 64 bits (8 bytes) representing the original message length in bits
uint64_t bits_len = 8 * initial_len;
// append 1 bit
uint64_t new_len = initial_len*8 + 1;
uint64_t new_len_bits = new_len % 512;
uint64_t append_len_bits;
if (new_len_bits <= (448)) {
append_len_bits = 448 - new_len_bits;
} else {
append_len_bits = 448 + (512 - new_len_bits);
}
uint64_t new_total_len = new_len + append_len_bits + 64;
msg = calloc(new_total_len / 8, 8);
memcpy(msg, initial_msg, initial_len);
msg[initial_len] = 128;
// Append message length, in bits, as 64-bit little-endian integer
msg[new_total_len - 8] = (bits_len & 0xff00000000000000) >> 56;
msg[new_total_len - 7] = (bits_len & 0x00ff000000000000) >> 48;
msg[new_total_len - 6] = (bits_len & 0x0000ff0000000000) >> 40;
msg[new_total_len - 5] = (bits_len & 0x000000ff00000000) >> 32;
msg[new_total_len - 4] = (bits_len & 0x00000000ff000000) >> 24;
msg[new_total_len - 3] = (bits_len & 0x0000000000ff0000) >> 16;
msg[new_total_len - 2] = (bits_len & 0x000000000000ff00) >> 8;
msg[new_total_len - 1] = (bits_len & 0x00000000000000ff);
// Process the message in successive 512-bit chunks:
// for each 512-bit chunk of message:
for (int i = 0; i < new_total_len; i += (512 / 8)) {
// break chunk into sixteen 32-bit words w[j], 0 ≤ j ≤ 15
uint32_t *w = (uint32_t *) (msg + i);
uint32_t a = h0;
uint32_t b = h1;
uint32_t c = h2;
uint32_t d = h3;
uint32_t f, g;
// Main loop:
for (int j = 0; j < 64; j++) {
if (j < 16) {
f = (b & c) | ((~b) & d);
g = j;
} else if (j < 32) {
f = (d & b) | ((~d) & c);
g = (5*j + 1) % 16;
} else if (j < 48) {
f = b ^ c ^ d;
g = (3*j + 5) % 16;
} else {
f = c ^ (b | (~d));
g = (7*j) % 16;
}
uint32_t temp = d;
d = c;
c = b;
b = b + LEFTROTATE((a + f + k[j] + w[g]), r[j]);
a = temp;
}
// Add this chunk's hash to result so far:
h0 += a;
h1 += b;
h2 += c;
h3 += d;
}
// cleanup
free(msg);
// digest = hash(a,b,c,d) (little-endian style)
digest[0] = (h0 & 0xff);
digest[1] = (h0 >> 8) & 0xff;
digest[2] = (h0 >> 16) & 0xff;
digest[3] = (h0 >> 24) & 0xff;
digest[4] = (h1 & 0xff);
digest[5] = (h1 >> 8) & 0xff;
digest[6] = (h1 >> 16) & 0xff;
digest[7] = (h1 >> 24) & 0xff;
digest[8] = (h2 & 0xff);
digest[9] = (h2 >> 8) & 0xff;
digest[10] = (h2 >> 16) & 0xff;
digest[11] = (h2 >> 24) & 0xff;
digest[12] = (h3 & 0xff);
digest[13] = (h3 >> 8) & 0xff;
digest[14] = (h3 >> 16) & 0xff;
digest[15] = (h3 >> 24) & 0xff;
}
int main() {
uint8_t digest[16];
char *msg1 = "hello world";
uint8_t *data1 = (uint8_t *)msg1;
size_t len1 = strlen(msg1);
md5(data1, len1, digest);
printf("Digest (MD5) of message '%s': ", msg1);
for(int i = 0; i < 16; i++) {
printf("%x", digest[i]);
}
printf("\n");
char *msg2 = "test123";
uint8_t *data2 = (uint8_t *)msg2;
size_t len2 = strlen(msg2);
md5(data2, len2, digest);
printf("Digest (MD5) of message '%s': ", msg2);
for(int i = 0; i < 16; i++) {
printf("%x", digest[i]);
}
printf("\n");
return 0;
}
```
这段代码实现了对给定字符串进行MD5加密的功能。可以根据需要修改代码,适应不同的使用场景。
阅读全文
相关推荐
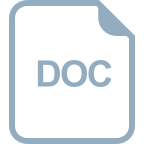
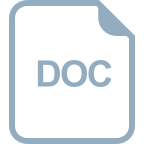
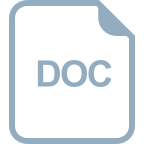
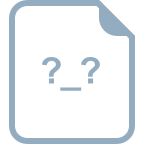
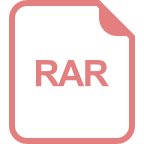
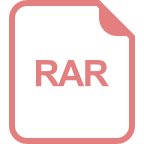
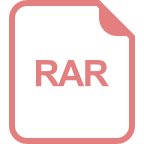
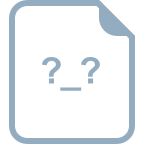
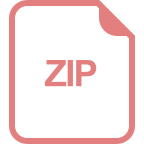
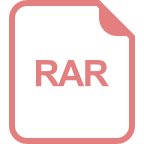
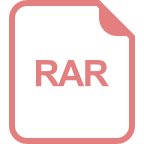
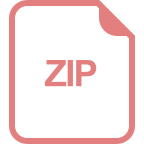
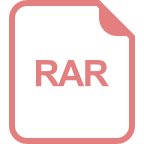
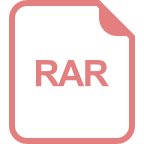

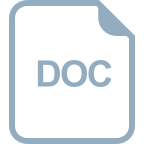