hashjoin 源码
时间: 2023-07-27 09:06:29 浏览: 63
由于 hash join 算法实现涉及到具体的数据库系统,不同数据库系统的 hash join 实现也会有所不同。这里以 PostgreSQL 数据库为例,简单介绍一下其 hash join 的实现。
在 PostgreSQL 中,hash join 算法主要分为两个阶段:构建哈希表和使用哈希表进行连接操作。
构建哈希表的过程中,首先会根据连接条件,从被连接关系中选择一个关系作为内部关系,并以内部关系的 join key 为基准进行哈希,将哈希值相同的元组放到一个桶中。这样,内部关系中的所有元组就被分配到了不同的桶中。接着,遍历外部关系中的元组,对于每个元组,都会计算其 join key 的哈希值,并在哈希表中查找是否有和该元组 join key 哈希值相同的桶。如果有,则将该元组与桶中的所有元组进行比较,找到符合连接条件的元组组合。如果没有,则该元组与内部关系中所有元组都没有匹配项,会被过滤掉。
构建好哈希表后,就可以使用哈希表进行连接操作了。遍历外部关系中的元组,对于每个元组,都计算其 join key 的哈希值,并在哈希表中查找是否有和该元组 join key 哈希值相同的桶。如果有,则将该元组与桶中的所有元组进行比较,找到符合连接条件的元组组合。如果没有,则该元组与内部关系中所有元组都没有匹配项,会被过滤掉。
以上就是 PostgreSQL 中哈希连接的实现过程,具体的源码实现可以参考 PostgreSQL 的源码。
相关推荐
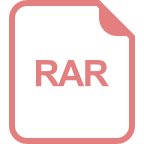
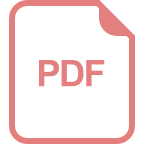
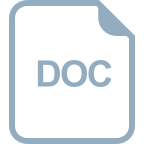
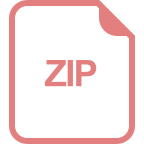
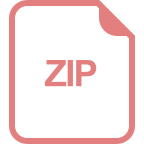
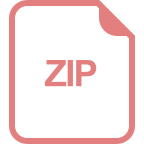
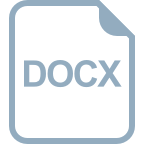
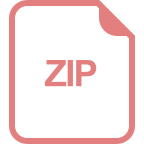
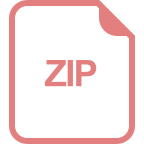
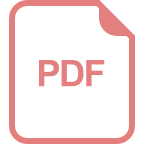
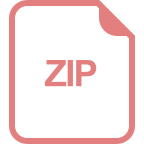
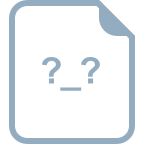
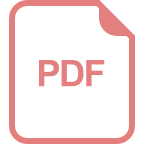