java给pdf加目录跳转
时间: 2023-07-20 14:25:26 浏览: 240
Java可以使用iText库来给PDF文件添加目录和跳转。具体操作步骤如下:
1. 首先,你需要在项目中引入iText库。你可以在maven仓库中搜索iText,然后将其添加到你的项目依赖中。
2. 然后,你需要创建一个PdfWriter对象和一个Document对象,用于将内容写入PDF文件。
```java
PdfWriter writer = new PdfWriter("output.pdf");
PdfDocument pdf = new PdfDocument(writer);
Document document = new Document(pdf);
```
3. 接着,你可以使用add方法将章节标题和内容添加到PDF文件中。同时,你需要使用add方法添加一个空白页,用于作为目录的起始页。
```java
document.add(new Paragraph("Chapter 1 - Introduction"));
document.add(new Paragraph("This is the introduction of the book."));
document.add(new AreaBreak());
document.add(new Paragraph("Chapter 2 - Getting Started"));
document.add(new Paragraph("This is the second chapter of the book."));
document.add(new AreaBreak());
```
4. 然后,你需要创建一个PdfOutline对象,用于添加目录项和跳转链接。
```java
PdfOutline root = pdf.getOutlines(false);
PdfOutline introduction = root.addOutline("Introduction");
introduction.addDestination(PdfDestination.makeDestination(new PdfString("Chapter 1 - Introduction")));
PdfOutline gettingStarted = root.addOutline("Getting Started");
gettingStarted.addDestination(PdfDestination.makeDestination(new PdfString("Chapter 2 - Getting Started")));
```
5. 最后,你需要关闭Document对象和PdfWriter对象,以便将内容写入PDF文件。
```java
document.close();
writer.close();
```
完整的示例代码如下:
```java
import com.itextpdf.io.font.FontConstants;
import com.itextpdf.kernel.pdf.PdfDocument;
import com.itextpdf.kernel.pdf.PdfDestination;
import com.itextpdf.kernel.pdf.PdfOutline;
import com.itextpdf.kernel.pdf.PdfString;
import com.itextpdf.kernel.pdf.PdfWriter;
import com.itextpdf.layout.Document;
import com.itextpdf.layout.element.AreaBreak;
import com.itextpdf.layout.element.Paragraph;
import java.io.IOException;
public class AddTableOfContentsToPDF {
public static void main(String[] args) throws IOException {
// Create a PdfWriter and a Document
PdfWriter writer = new PdfWriter("output.pdf");
PdfDocument pdf = new PdfDocument(writer);
Document document = new Document(pdf);
// Add chapter headings and content
document.add(new Paragraph("Chapter 1 - Introduction"));
document.add(new Paragraph("This is the introduction of the book."));
document.add(new AreaBreak());
document.add(new Paragraph("Chapter 2 - Getting Started"));
document.add(new Paragraph("This is the second chapter of the book."));
document.add(new AreaBreak());
// Add a table of contents
PdfOutline root = pdf.getOutlines(false);
PdfOutline introduction = root.addOutline("Introduction");
introduction.addDestination(PdfDestination.makeDestination(new PdfString("Chapter 1 - Introduction")));
PdfOutline gettingStarted = root.addOutline("Getting Started");
gettingStarted.addDestination(PdfDestination.makeDestination(new PdfString("Chapter 2 - Getting Started")));
// Close the Document and the PdfWriter
document.close();
writer.close();
}
}
```
这样,就可以在Java中使用iText库给PDF文件添加目录和跳转了。
阅读全文
相关推荐
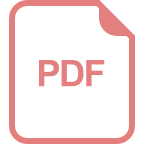




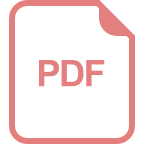
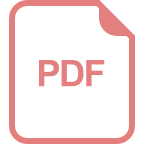
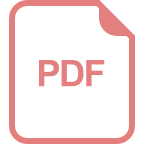
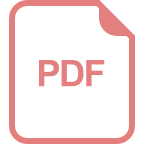
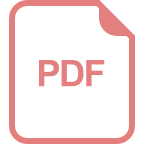





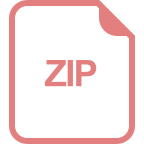
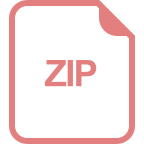