服务器上部署python,客户机上调用服务器上的python运行
时间: 2024-01-11 12:46:31 浏览: 137
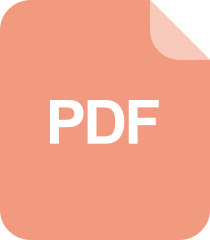
Python调用scp向服务器上传文件示例
要在客户机上调用服务器上的Python程序,可以使用远程过程调用(RPC)框架。其中一种流行的RPC框架是gRPC,它使用Protocol Buffers作为接口定义语言(IDL)和二进制协议。以下是一些基本步骤:
1. 在服务器上安装Python和gRPC Python库。
2. 使用gRPC编写服务器端代码,并将其部署到服务器上。
3. 在客户机上安装gRPC Python库。
4. 使用gRPC编写客户端代码,并将其在客户机上运行。
在客户端代码中,您需要指定服务器的IP地址和端口号,以便能够连接到服务器。然后,您可以使用gRPC提供的方法来调用服务器上的Python程序并接收结果。
下面是一个简单的示例,演示如何使用gRPC在客户端上调用名为"hello"的Python函数:
1. 首先,您需要定义接口和消息类型。这可以通过.proto文件完成,例如:
```protobuf
syntax = "proto3";
package hello;
service HelloService {
rpc SayHello (HelloRequest) returns (HelloResponse) {}
}
message HelloRequest {
string name = 1;
}
message HelloResponse {
string message = 1;
}
```
2. 然后,您可以使用gRPC编译器生成Python代码:
```
python -m grpc_tools.protoc -I. --python_out=. --grpc_python_out=. hello.proto
```
3. 在服务器端,您可以编写以下代码实现接口:
```python
import grpc
from concurrent import futures
import hello_pb2
import hello_pb2_grpc
class HelloService(hello_pb2_grpc.HelloServiceServicer):
def SayHello(self, request, context):
message = f"Hello, {request.name}!"
return hello_pb2.HelloResponse(message=message)
server = grpc.server(futures.ThreadPoolExecutor(max_workers=10))
hello_pb2_grpc.add_HelloServiceServicer_to_server(HelloService(), server)
server.add_insecure_port('[::]:50051')
server.start()
server.wait_for_termination()
```
4. 在客户端,您可以编写以下代码调用服务器上的"SayHello"方法:
```python
import grpc
import hello_pb2
import hello_pb2_grpc
channel = grpc.insecure_channel('localhost:50051')
stub = hello_pb2_grpc.HelloServiceStub(channel)
response = stub.SayHello(hello_pb2.HelloRequest(name='Alice'))
print(response.message)
```
当您在客户端上运行此代码时,它将连接到服务器上的50051端口,并调用"SayHello"方法。服务器将返回消息,客户端将打印出该消息,即“Hello, Alice!”
阅读全文
相关推荐
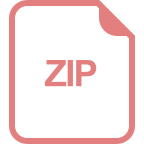
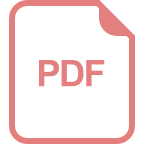















