在PyCharm中实现查询MySQL数据并输出
时间: 2024-03-05 14:49:33 浏览: 94
在 PyCharm 中查询 MySQL 数据并输出可以参考以下示例代码:
```python
import mysql.connector
mydb = mysql.connector.connect(
host="localhost",
user="yourusername",
password="yourpassword",
database="mydatabase"
)
mycursor = mydb.cursor()
mycursor.execute("SELECT * FROM customers")
myresult = mycursor.fetchall()
for x in myresult:
print(x)
```
在该示例中,我们从名为 `customers` 的表中检索了所有数据,并使用 `fetchall()` 方法将结果存储在 `myresult` 变量中。然后,通过循环遍历 `myresult` 变量并打印每个数据行。
如果需要将查询结果输出到文件中,可以使用以下代码:
```python
import mysql.connector
mydb = mysql.connector.connect(
host="localhost",
user="yourusername",
password="yourpassword",
database="mydatabase"
)
mycursor = mydb.cursor()
mycursor.execute("SELECT * FROM customers")
myresult = mycursor.fetchall()
with open('output.txt', 'w') as f:
for x in myresult:
f.write(str(x) + '\n')
```
在该示例中,我们将查询结果输出到名为 `output.txt` 的文件中。对于每个数据行,我们使用 `write()` 方法将其转换为字符串并写入文件中。
最后,当查询完成后,需要关闭数据库连接:
```python
mydb.close()
```
以上就是在 PyCharm 中查询 MySQL 数据并输出的基本步骤。
阅读全文
相关推荐
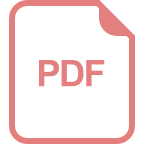
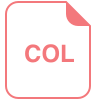
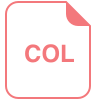
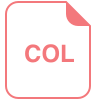
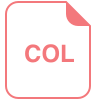
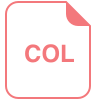
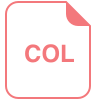
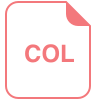
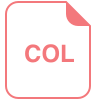
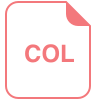
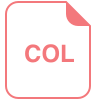
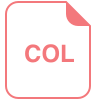




