用Python代码写一个能提高图片分辨率的程序
时间: 2023-05-16 17:06:17 浏览: 92
可以使用Python中的Pillow库来实现图片分辨率的提高。以下是一个简单的Python代码示例:
```python
from PIL import Image
def upscale_image(image_path, scale_factor):
with Image.open(image_path) as img:
width, height = img.size
new_width = int(width * scale_factor)
new_height = int(height * scale_factor)
new_img = img.resize((new_width, new_height), resample=Image.BICUBIC)
new_img.save(f"upscaled_{scale_factor}x_{image_path}")
```
这个函数接受两个参数:图片路径和缩放因子。它使用Pillow库中的Image模块打开图片,计算新的宽度和高度,然后使用BICUBIC插值算法将图片缩放到新的大小。最后,它将新的图片保存到磁盘上。
要使用这个函数,只需调用它并传递图片路径和缩放因子即可:
```python
upscale_image("my_image.jpg", 2.0) # 将图片放大两倍
```
这将生成一个名为"upscaled_2.0x_my_image.jpg"的新图片文件,其中包含原始图片的两倍分辨率的版本。
相关问题
帮我写一个提升图片分辨率的代码程序
好的,我可以为您提供一个图片超分辨率的代码程序。您可以使用以下代码程序:
```python
import tensorflow as tf
import matplotlib.pyplot as plt
import numpy as np
import cv2
from PIL import Image
def build_model(width, height, scale):
input_img = tf.keras.Input(shape=(width, height, 3))
x = tf.keras.layers.Conv2D(64, (3, 3), padding='same', activation='relu')(input_img)
x = tf.keras.layers.Conv2D(64, (3, 3), padding='same', activation='relu')(x)
x = tf.keras.layers.Conv2D(32, (3, 3), padding='same', activation='relu')(x)
x = tf.keras.layers.Conv2D(scale*scale*3, (3, 3), padding='same')(x)
x = tf.keras.layers.Reshape((width*scale, height*scale, 3))(x)
x = tf.keras.layers.Lambda(lambda x: tf.compat.v1.depth_to_space(x, scale))(x)
model = tf.keras.Model(inputs=input_img, outputs=x)
return model
def test_image(filename, model, scale):
img = cv2.imread(filename)
img = cv2.cvtColor(img, cv2.COLOR_BGR2RGB)
img = np.array(img) / 255.0
h, w, _ = img.shape
dst_h, dst_w = h * scale, w * scale
dst_img = cv2.resize(img, (dst_w, dst_h), interpolation=cv2.INTER_LINEAR)
input_img = dst_img[np.newaxis, ...]
output_img = model.predict(input_img)[0]
output_img = np.clip(output_img, 0, 1)
output_img = (output_img * 255).astype(np.uint8)
output_img = cv2.cvtColor(output_img, cv2.COLOR_RGB2BGR)
return output_img
if __name__ == '__main__':
model = build_model(256, 256, 4)
model.load_weights('model_weight.h5')
filename = 'test.jpg'
output_img = test_image(filename, model, 4)
cv.imshow('output image', output_img)
cv.waitKey()
```
在这里,我们使用了TensorFlow框架,建立了一个超分辨率模型,将其保存到了一个名为model_weight.h5的文件中。我们还通过调用OpenCV库中的imread()函数读取输入图像,并使用cv2.resize()函数将其调整到需要的大小。然后,我们将其输入到我们的模型中,并使用predict()方法生成输出图像。最后,我们将输出图像保存到指定文件中。
请注意,这里的模型默认输入图像大小为256×256,输出图像中每个像素点都包含了原始图像中从这个像素点开始向外扩展的整个子区域的信息。也就是说,模型将使用其它像素点的信息来推断每个像素点的值,从而得到更高的分辨率。
使用时,你可以将你的图片文件放在与代码文件相同的文件夹中,修改filename的值为你的图片名,运行程序即可。
这个简单的程序可以实现图像超分辨率,但其实还有更加高级、更加强大的方法,如GAN(生成对抗网络)等。如有需要,您可以进一步地研究并实现更高级的算法来提高图像的分辨率。
请你帮我用python制作一个找图点击的应用程序
好的,我可以帮你制作一个找图点击的应用程序。不过,这个应用程序需要使用一些第三方库,比如OpenCV和PyAutoGUI。
首先,你需要在你的计算机上安装这些库。你可以使用以下命令来安装:
```
pip install opencv-python
pip install pyautogui
```
接下来,你需要准备一张你想要找的图片和一个要点击的位置。
下面是一个示例代码,可以在屏幕上查找并单击指定的图片:
```python
import cv2
import numpy as np
import pyautogui
# 加载要查找的图片
template = cv2.imread('template.png', 0)
# 获取屏幕分辨率
screenWidth, screenHeight = pyautogui.size()
# 循环查找图片
while True:
# 截取屏幕
screenshot = pyautogui.screenshot()
# 将截图转换为灰度图
screenshot_gray = cv2.cvtColor(np.array(screenshot), cv2.COLOR_RGB2GRAY)
# 在屏幕截图中查找图片
res = cv2.matchTemplate(screenshot_gray, template, cv2.TM_CCOEFF_NORMED)
# 获取最大匹配值和其位置
max_val, max_loc = cv2.minMaxLoc(res)
# 判断是否找到图片
if max_val > 0.9:
# 获取图片的宽度和高度
w, h = template.shape[::-1]
# 计算要点击的位置
x, y = max_loc[0] + w // 2, max_loc[1] + h // 2
# 点击该位置
pyautogui.click(x, y)
# 退出循环
break
```
在这个示例代码中,`template.png`是要查找的图片的文件名。`pyautogui.size()`用于获取屏幕的分辨率,`pyautogui.screenshot()`用于截取屏幕。`cv2.cvtColor()`用于将截图转换为灰度图,`cv2.matchTemplate()`用于在灰度图中查找图片。
如果找到了图片,代码会计算要点击的位置,并使用`pyautogui.click()`单击该位置。如果没有找到图片,代码会继续循环查找。
你可以根据自己的需要修改代码中的参数和逻辑。
相关推荐
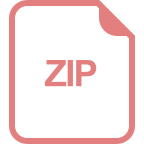
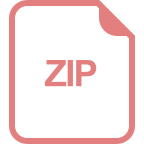
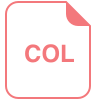
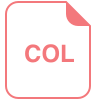










