要一个五个功能模块并且带有php后端的微信小程序
时间: 2024-04-01 17:32:31 浏览: 50
好的,这是一个带有五个功能模块并且带有 PHP 后端的微信小程序的代码示例。这个小程序包含了以下五个功能模块:
1. 首页:展示产品列表、广告轮播图等信息。
2. 分类页:按照产品类别展示产品列表。
3. 购物车:展示已选购的产品列表和总价。
4. 订单页:展示已下单的订单列表。
5. 个人中心:展示用户个人信息和订单列表。
小程序的后端使用 PHP 语言编写,使用 MySQL 数据库存储数据。以下是小程序的代码示例:
前端代码:
```html
<!-- 首页 -->
<view class="container">
<swiper autoplay circular interval="3000" duration="500">
<swiper-item wx:for="{{banners}}" wx:key="{{item.id}}">
<image src="{{item.src}}" mode="aspectFill"></image>
</swiper-item>
</swiper>
<scroll-view class="product-list" scroll-y="true">
<view wx:for="{{products}}" wx:key="{{item.id}}" class="product-item">
<image src="{{item.src}}" mode="aspectFill"></image>
<view class="product-info">
<view class="product-title">{{item.title}}</view>
<view class="product-price">{{item.price}}元</view>
<button class="add-cart-btn" bindtap="onAddCart" data-id="{{item.id}}">加入购物车</button>
</view>
</view>
</scroll-view>
</view>
<!-- 分类页 -->
<view class="container">
<scroll-view class="category-list" scroll-y="true">
<view wx:for="{{categories}}" wx:key="{{item.id}}" class="category-item" bindtap="onCategorySelected" data-id="{{item.id}}">
{{item.name}}
</view>
</scroll-view>
<scroll-view class="product-list" scroll-y="true">
<view wx:for="{{products}}" wx:key="{{item.id}}" class="product-item">
<image src="{{item.src}}" mode="aspectFill"></image>
<view class="product-info">
<view class="product-title">{{item.title}}</view>
<view class="product-price">{{item.price}}元</view>
<button class="add-cart-btn" bindtap="onAddCart" data-id="{{item.id}}">加入购物车</button>
</view>
</view>
</scroll-view>
</view>
<!-- 购物车 -->
<view class="container">
<scroll-view class="cart-list" scroll-y="true">
<view wx:for="{{cartItems}}" wx:key="{{item.id}}" class="cart-item">
<image src="{{item.src}}" mode="aspectFill"></image>
<view class="cart-info">
<view class="cart-title">{{item.title}}</view>
<view class="cart-price">{{item.price}}元</view>
<button class="remove-cart-btn" bindtap="onRemoveCart" data-id="{{item.id}}">删除</button>
</view>
</view>
</scroll-view>
<view class="cart-total">
<view class="cart-total-label">总价:</view>
<view class="cart-total-price">{{totalPrice}}元</view>
<button class="checkout-btn" bindtap="onCheckout">结算</button>
</view>
</view>
<!-- 订单页 -->
<view class="container">
<scroll-view class="order-list" scroll-y="true">
<view wx:for="{{orders}}" wx:key="{{item.id}}" class="order-item">
<view class="order-info">
<view class="order-id">{{item.id}}</view>
<view class="order-price">{{item.price}}元</view>
</view>
<view class="order-status">{{item.status}}</view>
</view>
</scroll-view>
</view>
<!-- 个人中心 -->
<view class="container">
<view class="user-info">
<image src="{{userInfo.avatarUrl}}" mode="aspectFill"></image>
<view class="user-name">{{userInfo.nickName}}</view>
</view>
<scroll-view class="order-list" scroll-y="true">
<view wx:for="{{orders}}" wx:key="{{item.id}}" class="order-item">
<view class="order-info">
<view class="order-id">{{item.id}}</view>
<view class="order-price">{{item.price}}元</view>
</view>
<view class="order-status">{{item.status}}</view>
</view>
</scroll-view>
</view>
```
后端代码:
```php
<?php
// 连接数据库
$conn = mysqli_connect("localhost", "root", "password", "database_name");
// 获取产品列表
function getProducts() {
global $conn;
$result = mysqli_query($conn, "SELECT * FROM products");
$products = mysqli_fetch_all($result, MYSQLI_ASSOC);
return $products;
}
// 获取分类列表
function getCategories() {
global $conn;
$result = mysqli_query($conn, "SELECT * FROM categories");
$categories = mysqli_fetch_all($result, MYSQLI_ASSOC);
return $categories;
}
// 获取购物车列表
function getCartItems() {
global $conn;
$result = mysqli_query($conn, "SELECT * FROM cart_items");
$cartItems = mysqli_fetch_all($result, MYSQLI_ASSOC);
return $cartItems;
}
// 添加购物车项
function addCartItem($productId) {
global $conn;
mysqli_query($conn, "INSERT INTO cart_items (product_id) VALUES ($productId)");
}
// 删除购物车项
function removeCartItem($id) {
global $conn;
mysqli_query($conn, "DELETE FROM cart_items WHERE id = $id");
}
// 获取订单列表
function getOrders() {
global $conn;
$result = mysqli_query($conn, "SELECT * FROM orders");
$orders = mysqli_fetch_all($result, MYSQLI_ASSOC);
return $orders;
}
// 下订单
function placeOrder($cartItems, $totalPrice) {
global $conn;
mysqli_query($conn, "INSERT INTO orders (items, price) VALUES ('$cartItems', $totalPrice)");
}
// 获取用户信息
function getUserInfo($openId) {
global $conn;
$result = mysqli_query($conn, "SELECT * FROM users WHERE open_id = '$openId'");
$userInfo = mysqli_fetch_assoc($result);
return $userInfo;
}
```
以上只是示例代码,实际使用中你需要根据自己的需求进行修改和完善。
阅读全文
相关推荐
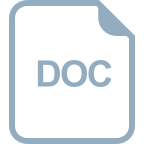
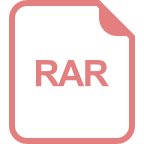
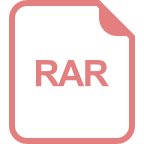
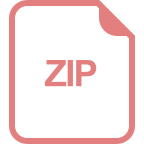
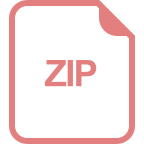
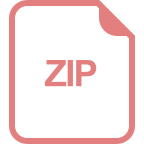
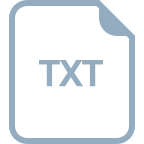
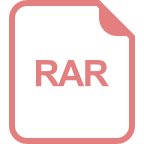
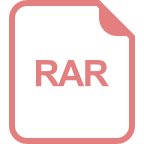
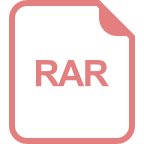
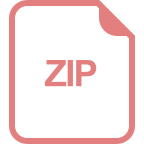
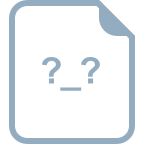
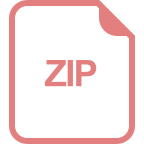
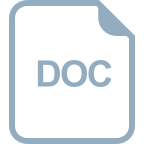
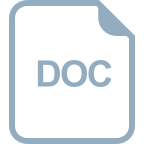
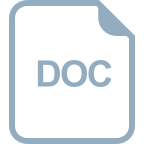