android 文件上传进度
时间: 2023-08-04 13:33:51 浏览: 145
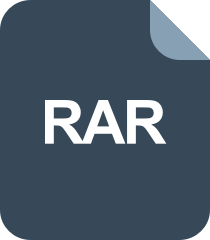
Android 文件上传 带上传进度

在 Android 中进行文件上传并显示上传进度的一种常见方式是使用 OkHttp 库。具体实现步骤如下:
1. 添加 OkHttp 库依赖:在 app 的 build.gradle 文件中添加以下代码:
```gradle
dependencies {
implementation 'com.squareup.okhttp3:okhttp:4.9.0'
}
```
2. 创建一个 RequestBody 对象,该对象包含将要上传的文件内容。
```java
File file = new File(filePath);
RequestBody requestBody = RequestBody.create(MediaType.parse("multipart/form-data"), file);
```
3. 创建一个 ProgressRequestBody 对象,该对象可以监听请求的上传进度。
```java
ProgressRequestBody progressRequestBody = new ProgressRequestBody(requestBody, new ProgressRequestBody.Listener() {
@Override
public void onProgressChanged(long numBytes, long totalBytes, float percent) {
// 在这里更新上传进度
}
});
```
4. 创建一个 MultipartBody.Part 对象,该对象将上传的文件内容包装成一个“Part”。
```java
MultipartBody.Part part = MultipartBody.Part.createFormData("file", file.getName(), progressRequestBody);
```
5. 创建一个 Request 对象,该对象包含上传文件的 URL 和其他参数。
```java
Request request = new Request.Builder()
.url(uploadUrl)
.post(part)
.build();
```
6. 使用 OkHttp 发送请求,并在 onResponse 回调中处理上传结果。
```java
OkHttpClient client = new OkHttpClient();
client.newCall(request).enqueue(new Callback() {
@Override
public void onFailure(Call call, IOException e) {
// 上传失败
}
@Override
public void onResponse(Call call, Response response) throws IOException {
// 上传成功
}
});
```
在上面的代码中,我们自定义了一个 ProgressRequestBody 类来监听上传进度。该类的实现如下:
```java
public class ProgressRequestBody extends RequestBody {
private final RequestBody requestBody;
private final Listener listener;
public ProgressRequestBody(RequestBody requestBody, Listener listener) {
this.requestBody = requestBody;
this.listener = listener;
}
@Override
public MediaType contentType() {
return requestBody.contentType();
}
@Override
public long contentLength() throws IOException {
return requestBody.contentLength();
}
@Override
public void writeTo(BufferedSink sink) throws IOException {
BufferedSink bufferedSink = Okio.buffer(new ForwardingSink(sink));
requestBody.writeTo(bufferedSink);
bufferedSink.flush();
}
public interface Listener {
void onProgressChanged(long numBytes, long totalBytes, float percent);
}
private class ForwardingSink extends ForwardingSink {
private long numBytesWritten = 0L;
private long totalBytes = 0L;
public ForwardingSink(Sink delegate) {
super(delegate);
try {
totalBytes = contentLength();
} catch (IOException e) {
e.printStackTrace();
}
}
@Override
public void write(Buffer source, long byteCount) throws IOException {
super.write(source, byteCount);
numBytesWritten += byteCount;
listener.onProgressChanged(numBytesWritten, totalBytes, numBytesWritten / (float) totalBytes);
}
}
}
```
在 ForwardingSink 类中,我们通过调用 listener.onProgressChanged() 方法来通知上传进度的变化。通过在 onProgressChanged() 方法中更新 UI 来显示上传进度即可。
阅读全文
相关推荐
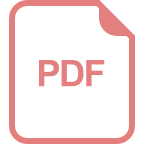
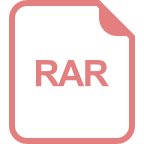
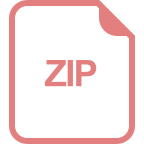
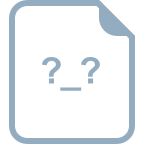
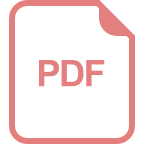
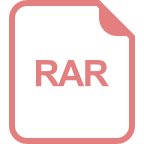
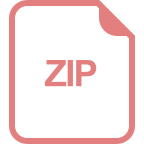
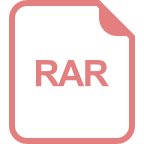
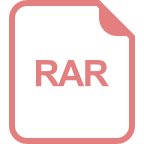
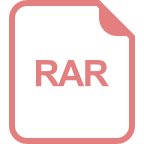
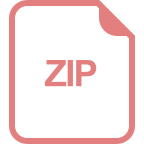
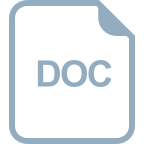
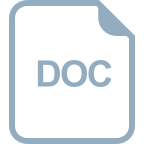
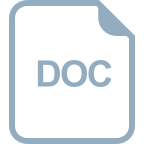
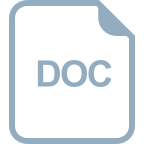