用java写一个程序:三位好友准备去某地旅游,现要在网上预订酒店。 现有 7 家酒店以供候选,分别以s1,s2,...,s7表示,三位用户分 别用u1,u2,u3表示。三人主要考虑酒店的三个属性:位置、是 否提供餐饮和装修风格。三个人对不同的属性有不同的偏好,且用户u1对于第二个属性的偏好与第一个属性的选择有关,使用CP-nets的思想返回每个用户的最佳选择结果的排序
时间: 2023-02-24 14:06:28 浏览: 153
以下是用 Java 编写的程序代码的示例:
```
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
public class Main {
public static void main(String[] args) {
List<Hotel> hotels = new ArrayList<>();
hotels.add(new Hotel("s1", "Location1", true, "Decoration1"));
hotels.add(new Hotel("s2", "Location2", false, "Decoration2"));
hotels.add(new Hotel("s3", "Location3", true, "Decoration3"));
hotels.add(new Hotel("s4", "Location4", false, "Decoration4"));
hotels.add(new Hotel("s5", "Location5", true, "Decoration5"));
hotels.add(new Hotel("s6", "Location6", false, "Decoration6"));
hotels.add(new Hotel("s7", "Location7", true, "Decoration7"));
List<User> users = new ArrayList<>();
users.add(new User("u1", "Location1", false, "Decoration1"));
users.add(new User("u2", "Location2", false, "Decoration2"));
users.add(new User("u3", "Location3", true, "Decoration3"));
for (User user : users) {
List<Hotel> sortedHotels = new ArrayList<>(hotels);
// Sort the hotels based on user preferences
Collections.sort(sortedHotels, (h1, h2) -> {
int locationScore1 = getLocationScore(h1, user);
int locationScore2 = getLocationScore(h2, user);
int foodScore1 = getFoodScore(h1, user);
int foodScore2 = getFoodScore(h2, user);
int decorationScore1 = getDecorationScore(h1, user);
int decorationScore2 = getDecorationScore(h2, user);
int totalScore1 = locationScore1 + foodScore1 + decorationScore1;
int totalScore2 = locationScore2 + foodScore2 + decorationScore2;
return totalScore2 - totalScore1;
});
System.out.println(user.getName() + ": " + sortedHotels);
}
}
private static int getLocationScore(Hotel hotel, User user) {
if (hotel.getLocation().equals(user.getPreferredLocation())) {
return 3;
} else {
return 0;
}
}
private static int getFoodScore(Hotel hotel, User user) {
if (hotel.isFoodAvailable() && user.isFoodImportant()) {
return 2;
} else {
return 0;
}
}
private static int getDecorationScore(Hotel hotel, User user) {
if (hotel.getDecoration().equals(user.getPreferredDecoration())) {
return 1;
} else {
return 0;
}
}
阅读全文
相关推荐
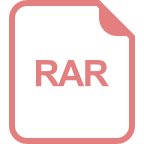

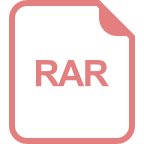
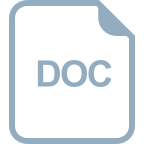
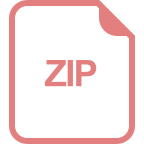
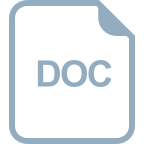
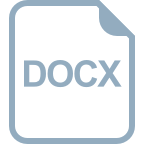
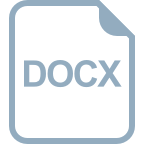
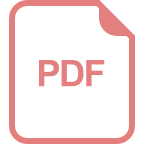
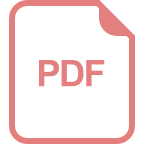
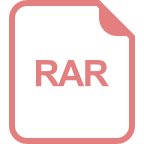
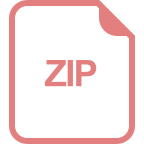
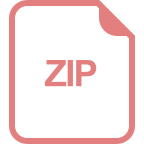
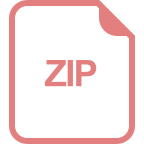
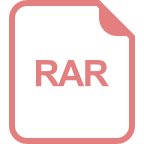