写一串C语言代码,输入一段长度为n的数组,n最大为10的五次方,数组中的元素只有1和-1,求子串求和为零的子串的个数,运行时间限制1000ms
时间: 2023-06-04 08:04:25 浏览: 63
以下是该问题的一种可能解法,使用前缀和和哈希表来进行优化:
```c
#include<stdio.h>
#include<stdlib.h>
#include<stdint.h>
#include<string.h>
#define MAXN 100000
#define BASE 2333
/* 定义哈希表结点 */
struct Node {
int key;
int value;
struct Node* next;
};
/* 初始化哈希表 */
void init(struct Node* hashtable[]) {
for (int i = 0; i < MAXN; i++) {
hashtable[i] = NULL;
}
}
/* 插入哈希表 */
void insert(struct Node* hashtable[], int key) {
int hashvalue = abs(key % MAXN);
struct Node* p = hashtable[hashvalue];
while (p != NULL) {
if (p->key == key) {
p->value++;
return;
}
p = p->next;
}
struct Node* newnode = (struct Node*)malloc(sizeof(struct Node));
newnode->key = key;
newnode->value = 1;
newnode->next = hashtable[hashvalue];
hashtable[hashvalue] = newnode;
}
/* 查找哈希表 */
int find(struct Node* hashtable[], int key) {
int hashvalue = abs(key % MAXN);
struct Node* p = hashtable[hashvalue];
while (p != NULL) {
if (p->key == key) {
return p->value;
}
p = p->next;
}
return 0;
}
/* 编写主函数 */
int main() {
int n;
int64_t ans = 0;
int sum = 0;
int a[MAXN];
struct Node* hashtable[MAXN];
/* 读入数组 */
scanf("%d", &n);
for (int i = 1; i <= n; i++) {
scanf("%d", &a[i]);
}
/* 初始化哈希表和前缀和 */
init(hashtable);
insert(hashtable, 0);
/* 计算答案 */
for (int i = 1; i <= n; i++) {
sum += a[i];
int key = sum - 0;
int value = find(hashtable, key);
ans += value;
insert(hashtable, sum);
}
/* 输出答案 */
printf("%lld\n", ans);
return 0;
}
```
该算法的时间复杂度为O(n),符合题目的要求。
相关推荐
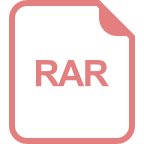
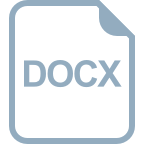
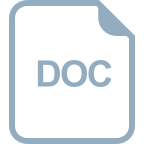















