cluster_path = os.path.join(path, cluster_folder) if not os.path.exists(cluster_path): os.mkdir(cluster_path) for i in range(10): cluster_i_path = os.path.join(cluster_path, str(i)) if not os.path.exists(cluster_i_path): os.mkdir(cluster_i_path) for j, label in enumerate(labels): if label == i: old_path = os.path.join(path, f'{j}.png') new_path = os.path.join(cluster_i_path, f'{j}.png') os.rename(old_path, new_path),这段代码是什么意思
时间: 2024-04-28 09:23:29 浏览: 135
这段代码的作用是将指定路径下的图像文件按照分类标签进行聚类,并将聚类结果保存到不同的文件夹中。
具体来说,该代码首先通过 `os.path.join()` 函数将 `path` 和 `cluster_folder` 拼接成完整的聚类文件夹路径 `cluster_path`,然后使用 `os.mkdir()` 函数创建聚类文件夹,如果该文件夹已经存在则不进行任何操作。
接着,该代码使用一个循环对每个聚类标签进行处理。对于每个标签,它会创建一个以标签名称命名的子文件夹,并使用 `os.mkdir()` 函数创建该文件夹,如果该文件夹已经存在则不进行任何操作。
最后,该代码使用另一个循环对每个图像文件进行处理。对于每个文件,它会根据文件对应的标签将其移动到对应的聚类文件夹中,移动操作使用 `os.rename()` 函数实现。具体来说,它首先构造出旧的文件路径 `old_path`,然后构造出新的文件路径 `new_path`,其中新路径包含聚类文件夹和文件名。最后,它使用 `os.rename()` 函数将旧的文件路径改名为新的文件路径,从而完成了文件的移动操作。
相关问题
# 将图片移动到相应的文件夹 cluster_path = os.path.join(path, cluster_folder) if not os.path.exists(cluster_path): os.mkdir(cluster_path) for i in range(10): cluster_i_path = os.path.join(cluster_path, str(i)) if not os.path.exists(cluster_i_path): os.mkdir(cluster_i_path) for j, label in enumerate(labels): if label == i: old_path = os.path.join(path, f'{j}.png') new_path = os.path.join(cluster_i_path, f'{j}.png') os.rename(old_path, new_path) root_path = r'D:\jk' for i in range(10): cluster_i_path = os.path.join(root_path, f'cluster_{i}') if not os.path.exists(cluster_i_path): os.mkdir(cluster_i_path) for j, label in enumerate(labels): if label == i: old_path = os.path.join(path, f'{j}.png') new_path = os.path.join(cluster_i_path, f'{j}.png') os.rename(old_path, new_path),这段代码有什么错误吗
这段代码没有语法错误,但是注意到两个循环中的变量 `i` 和 `j` 是一样的,所以在第二个循环中可以使用之前定义的变量 `i`,而不需要重新定义变量。修改后的代码如下:
```
root_path = r'D:\jk'
for i in range(10):
cluster_i_path = os.path.join(root_path, f'cluster_{i}')
if not os.path.exists(cluster_i_path):
os.mkdir(cluster_i_path)
for j, label in enumerate(labels):
if label == i:
old_path = os.path.join(path, f'{j}.png')
new_path = os.path.join(cluster_i_path, f'{j}.png')
os.rename(old_path, new_path)
```
另外,你需要将聚类文件夹的根目录 `root_path` 修改成你想要的路径。
D:\wjd这个路径下有2000多张1000*1000的png格式的ovito三色图片,现在想找用无监督方法对其自动分类,resnet50模型训练,不用k-means方法分类,然后在这个路径下D:\wjd建立文件夹,把特征相同的图片放在同一个文件夹里,
这个任务可以分为以下几个步骤:
1. 加载数据:使用Python中的PIL库或OpenCV库来读取图片,并将它们转换为numpy数组。
2. 特征提取:使用预训练的ResNet50模型来提取每张图片的特征向量。可以使用Keras中的ResNet50模型,并将其前一层作为特征提取器。
3. 无监督聚类:使用聚类算法将所有图片分为不同的类别。可以使用层次聚类、DBSCAN等无监督聚类算法。这里以层次聚类为例。
4. 将图片移动到相应的文件夹:根据聚类结果,将相同类别的图片移动到同一文件夹中。
下面是一个可能的实现代码:
```python
import os
import numpy as np
from PIL import Image
from keras.applications.resnet50 import ResNet50, preprocess_input
from sklearn.cluster import AgglomerativeClustering
# 定义路径和文件夹名称
path = 'D:/wjd'
feature_folder = 'features'
cluster_folder = 'clusters'
# 加载ResNet50模型
model = ResNet50(weights='imagenet', include_top=False, pooling='avg')
# 定义一个函数,用于提取每张图片的特征向量
def extract_features(img_path):
img = Image.open(img_path).resize((224, 224))
img_array = np.array(img)
img_array = np.expand_dims(img_array, axis=0)
img_array = preprocess_input(img_array)
features = model.predict(img_array)
return features.flatten()
# 遍历文件夹,提取特征向量
features = []
for filename in os.listdir(path):
if filename.endswith('.png'):
img_path = os.path.join(path, filename)
features.append(extract_features(img_path))
# 将特征向量保存到文件夹中
features_path = os.path.join(path, feature_folder)
if not os.path.exists(features_path):
os.mkdir(features_path)
np.save(os.path.join(features_path, 'features.npy'), np.array(features))
# 无监督聚类
clustering = AgglomerativeClustering(n_clusters=10)
labels = clustering.fit_predict(features)
# 将图片移动到相应的文件夹
cluster_path = os.path.join(path, cluster_folder)
if not os.path.exists(cluster_path):
os.mkdir(cluster_path)
for i in range(10):
cluster_i_path = os.path.join(cluster_path, str(i))
if not os.path.exists(cluster_i_path):
os.mkdir(cluster_i_path)
for j, label in enumerate(labels):
if label == i:
old_path = os.path.join(path, f'{j}.png')
new_path = os.path.join(cluster_i_path, f'{j}.png')
os.rename(old_path, new_path)
```
这段代码中,我们首先加载了ResNet50模型,并定义了一个函数`extract_features`,用于提取每张图片的特征向量。然后,我们遍历文件夹中的所有图片,提取它们的特征向量,并将这些向量保存到文件夹中。接着,使用层次聚类算法对这些特征向量进行聚类,并将相同类别的图片移动到同一文件夹中。最终,我们得到了一个按照特征相似度自动分类的结果。
阅读全文
相关推荐
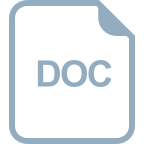
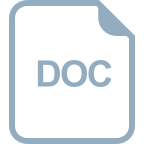
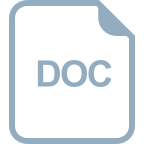





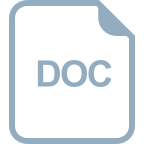
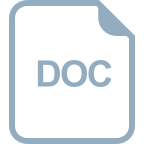
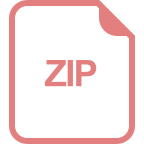
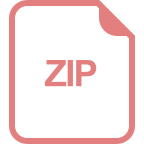
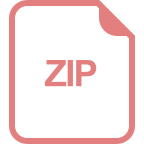
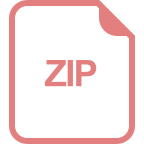
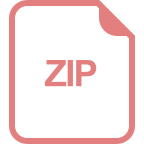
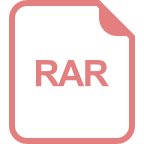
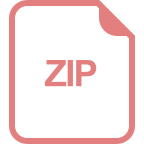