编写程序,使用文本文件将斐波那契数列前40个数保存在fib.txt文件中。然后打开这个文件,依次用数列的前一项除以后一项,将除的结果保存在fibst.txt中。打开文件观察结果。再用二进制文件的方式重新实现一遍,打开文件观察结果。
时间: 2024-02-09 07:10:21 浏览: 18
下面是使用文本文件和二进制文件分别实现的示例代码:
使用文本文件:
```c++
#include <iostream>
#include <fstream>
using namespace std;
int main() {
// 将斐波那契数列前40个数保存在文件中
ofstream fout("fib.txt");
int a = 0, b = 1, c;
fout << a << " " << b << " ";
for (int i = 2; i < 40; i++) {
c = a + b;
fout << c << " ";
a = b;
b = c;
}
fout.close();
// 读取文件中的斐波那契数列,计算相邻两数的商并保存在文件中
ifstream fin("fib.txt");
fout.open("fibst.txt");
int x, y;
fin >> x;
while (fin >> y) {
fout << (double)x / y << " ";
x = y;
}
fin.close();
fout.close();
return 0;
}
```
使用二进制文件:
```c++
#include <iostream>
#include <fstream>
using namespace std;
int main() {
// 将斐波那契数列前40个数保存在文件中
ofstream fout("fib.bin", ios::binary);
int a = 0, b = 1, c;
fout.write((char*)&a, sizeof(int));
fout.write((char*)&b, sizeof(int));
for (int i = 2; i < 40; i++) {
c = a + b;
fout.write((char*)&c, sizeof(int));
a = b;
b = c;
}
fout.close();
// 读取文件中的斐波那契数列,计算相邻两数的商并保存在文件中
ifstream fin("fib.bin", ios::binary);
fout.open("fibst.bin", ios::binary);
int x, y;
fin.read((char*)&x, sizeof(int));
while (fin.read((char*)&y, sizeof(int))) {
fout.write((char*)&((double)x / y), sizeof(double));
x = y;
}
fin.close();
fout.close();
return 0;
}
```
以上两段代码实现了将斐波那契数列前40个数保存在文件中,并计算相邻两数的商并保存在另一个文件中。其中,使用文本文件时,将整数和浮点数都以字符串的形式保存,使用空格分隔;使用二进制文件时,将整数和浮点数以二进制形式直接写入文件中,需要使用ios::binary模式打开文件。
相关推荐
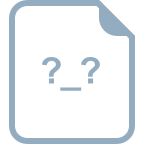











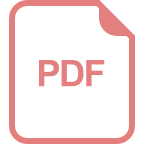
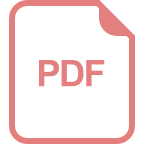
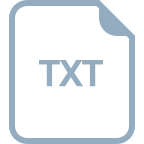