用c++写一个单词填空,给定中文释义和部分字母的代码
时间: 2023-12-03 16:43:06 浏览: 132
以下是一个简单的示例程序,可以让用户根据给定的中文释义和部分字母填写单词。
```c++
#include <iostream>
#include <string>
using namespace std;
int main() {
// 给定的中文释义和部分字母
string definition = "一种动物,有长长的脖子和腿,可以伸长到高处的树枝上吃树叶。";
string letters = "g_r__e_";
// 题目答案
string answer = "giraffe";
// 输出题目
cout << "请填写下列单词:" << endl;
cout << definition << endl;
cout << letters << endl;
// 用户输入答案
string userAnswer;
cin >> userAnswer;
// 判断用户答案是否正确
if (userAnswer == answer) {
cout << "恭喜你,回答正确!" << endl;
} else {
cout << "很遗憾,回答错误。正确答案是:" << answer << endl;
}
return 0;
}
```
程序会先输出给定的中文释义和部分字母,然后让用户输入答案。最后判断用户答案是否正确,并输出相应结果。
相关问题
编写c++程序开发一个单词记忆和考核系统。具有如下基本功能:支持文件导入中英文词典导入能够滚动播放单词,并且设定播放总时长和播放间隔能设定词汇范围(四六级、考研、托福)能进行如下方式的考核:听写单词填空,给定中文释义,和部分字母中英翻译(选择题)能记录每次考核的情况,能记录考核错误单词,并优先选择错误单词能记录个人使用轨迹,如使用时长,单词范围等,给设计鼓励体系,在不同的阶段颁发不同的鼓励勋章。
以下是一个简单的实现,仅供参考:
```cpp
#include <iostream>
#include <fstream>
#include <string>
#include <vector>
#include <ctime>
#include <cstdlib>
using namespace std;
// 单词类
class Word {
public:
Word(string e, string c): english(e), chinese(c) {}
string getEnglish() const { return english; }
string getChinese() const { return chinese; }
private:
string english;
string chinese;
};
// 单词表类
class WordList {
public:
WordList(string fileName) {
ifstream infile(fileName.c_str());
string english, chinese;
while (infile >> english >> chinese) {
words.push_back(Word(english, chinese));
}
infile.close();
}
int size() const { return words.size(); }
Word& operator[](int i) { return words[i]; }
private:
vector<Word> words;
};
// 考核类
class Exam {
public:
Exam(const WordList& wl): wordList(wl) {}
void listen() {
srand(time(NULL));
int numWords = wordList.size();
int totalTime, interval;
cout << "请输入播放总时长和播放间隔(秒):";
cin >> totalTime >> interval;
time_t start = time(NULL);
while (time(NULL) - start < totalTime) {
int index = rand() % numWords;
cout << wordList[index].getEnglish() << endl;
sleep(interval);
}
}
void fillBlank() {
int numWords = wordList.size();
int numRight = 0;
vector<int> wrongWords;
for (int i = 0; i < numWords; i++) {
cout << "请填写 \"" << wordList[i].getEnglish() << "\" 的中文翻译:";
string answer;
cin >> answer;
if (answer == wordList[i].getChinese()) {
cout << "回答正确!" << endl;
numRight++;
} else {
cout << "回答错误!正确答案为 \"" << wordList[i].getChinese() << "\"。" << endl;
wrongWords.push_back(i);
}
}
cout << "本次测试共 " << numWords << " 道题,你答对了 " << numRight << " 道,正确率为 " << (double)numRight / numWords * 100 << "%" << endl;
if (!wrongWords.empty()) {
cout << "以下是你答错的单词:" << endl;
for (int i = 0; i < wrongWords.size(); i++) {
cout << wordList[wrongWords[i]].getEnglish() << " ";
}
cout << endl;
}
}
void choice() {
int numWords = wordList.size();
int numRight = 0;
vector<int> wrongWords;
for (int i = 0; i < numWords; i++) {
cout << "请选择 \"" << wordList[i].getEnglish() << "\" 的中文翻译(输入数字):" << endl;
string choices[4];
int correctIndex = rand() % 4;
int wrongIndex = 0;
choices[correctIndex] = wordList[i].getChinese();
for (int j = 0; j < 4; j++) {
if (j == correctIndex) continue;
int index;
do {
index = rand() % numWords;
} while (index == i || index == wrongIndex);
choices[j] = wordList[index].getChinese();
wrongIndex = index;
}
for (int j = 0; j < 4; j++) {
cout << j + 1 << ". " << choices[j] << endl;
}
int answer;
cin >> answer;
if (answer == correctIndex + 1) {
cout << "回答正确!" << endl;
numRight++;
} else {
cout << "回答错误!正确答案为 \"" << wordList[i].getChinese() << "\"。" << endl;
wrongWords.push_back(i);
}
}
cout << "本次测试共 " << numWords << " 道题,你答对了 " << numRight << " 道,正确率为 " << (double)numRight / numWords * 100 << "%" << endl;
if (!wrongWords.empty()) {
cout << "以下是你答错的单词:" << endl;
for (int i = 0; i < wrongWords.size(); i++) {
cout << wordList[wrongWords[i]].getEnglish() << " ";
}
cout << endl;
}
}
private:
const WordList& wordList;
};
// 主函数
int main() {
string fileName;
cout << "请输入词典文件名:";
cin >> fileName;
WordList wordList(fileName);
Exam exam(wordList);
while (true) {
cout << "请选择操作:" << endl;
cout << "1. 滚动播放单词" << endl;
cout << "2. 听写单词填空" << endl;
cout << "3. 给定中文释义" << endl;
cout << "4. 部分字母中英翻译(选择题)" << endl;
cout << "5. 退出程序" << endl;
int choice;
cin >> choice;
switch (choice) {
case 1:
exam.listen();
break;
case 2:
exam.fillBlank();
break;
case 3:
exam.choice();
break;
case 4:
cout << "该功能暂未实现!" << endl;
break;
case 5:
cout << "程序已退出!" << endl;
return 0;
default:
cout << "无效操作,请重新输入!" << endl;
break;
}
}
return 0;
}
```
阅读全文
相关推荐
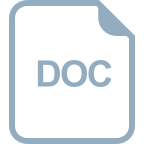
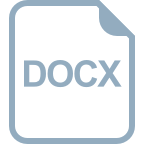
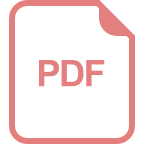
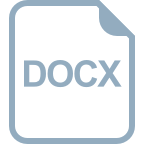
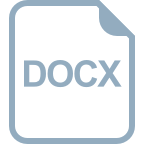
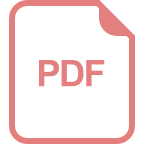
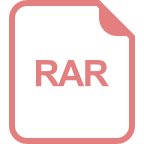
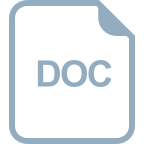
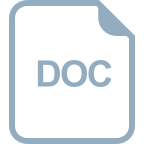
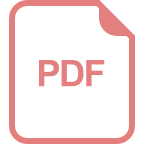
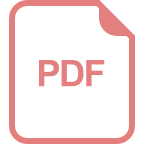
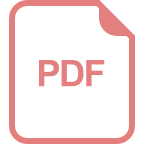
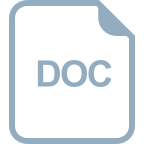