找出数组中的最大值,同时找出该最大值在数组中出现的次数。要求只能扫描一-次数组。
时间: 2023-09-14 09:05:28 浏览: 101
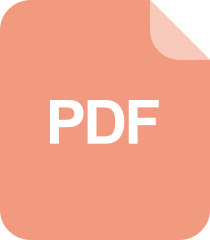
js求数组中全部数字可拼接出的最大整数示例代码
### 回答1:
可以使用一个变量来记录最大值,一个变量来记录最大值出现的次数。遍历数组,如果当前元素大于最大值,则更新最大值和最大值出现的次数为1;如果当前元素等于最大值,则最大值出现的次数加1。最后返回最大值和最大值出现的次数即可。
以下是示例代码:
```python
def find_max_and_count(arr):
max_val = arr[0]
max_count = 1
for i in range(1, len(arr)):
if arr[i] > max_val:
max_val = arr[i]
max_count = 1
elif arr[i] == max_val:
max_count += 1
return max_val, max_count
```
如果数组中有多个最大值,则返回其中任意一个最大值和其出现的次数。
### 回答2:
要找出数组中的最大值,同时统计该最大值在数组中出现的次数,可以通过扫描一次数组来实现。
首先初始化两个变量,maxValue用于记录最大值,count用于记录最大值出现的次数,初始值分别为数组的第一个元素和1。
然后使用循环遍历数组,从第二个元素开始,比较当前元素与maxValue的大小,如果当前元素大于maxValue,则更新maxValue为当前元素,并将count重置为1;如果当前元素等于maxValue,则count加1。
最后循环结束后,maxValue即为数组中的最大值,count即为最大值在数组中出现的次数。
下面是具体的代码实现:
```python
def findMaxValue(arr):
maxValue = arr[0]
count = 1
for i in range(1, len(arr)):
if arr[i] > maxValue:
maxValue = arr[i]
count = 1
elif arr[i] == maxValue:
count += 1
return maxValue, count
```
例如,对于数组[1, 5, 3, 5, 2, 5, 4],使用上述函数可以找到最大值为5,它在数组中出现了3次。
### 回答3:
要解决这个问题,可以使用如下的算法:
1. 假设初始最大值max为数组中的第一个元素,计数器count初始为1。
2. 从数组的第二个元素开始遍历,比较当前元素与最大值max的大小:
- 如果当前元素大于max,则更新max为当前元素,计数器count重置为1。
- 如果当前元素等于max,则计数器count加1。
- 如果当前元素小于max,则不进行任何操作。
3. 遍历完整个数组后,最大值max就是数组中的最大值,计数器count就是最大值在数组中出现的次数。
以下是一个示例代码实现:
```python
def find_max_and_count(arr):
max_value = arr[0]
count = 1
for i in range(1, len(arr)):
if arr[i] > max_value:
max_value = arr[i]
count = 1
elif arr[i] == max_value:
count += 1
return max_value, count
# 测试
array = [5, 2, 7, 3, 7, 7, 1]
max_value, count = find_max_and_count(array)
print("数组中的最大值为:", max_value)
print("最大值在数组中出现的次数为:", count)
```
运行以上代码的输出为:
```
数组中的最大值为: 7
最大值在数组中出现的次数为: 3
```
该算法只需要一次扫描数组,时间复杂度为O(n),其中n为数组的长度。
阅读全文
相关推荐
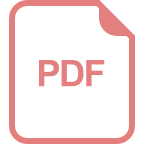
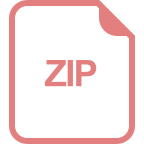














