帮我用html css JavaScript写一个能生成文件的日记网站
时间: 2024-06-11 08:07:18 浏览: 17
很高兴为您提供帮助。以下是一个简单的日记网站示例,您可以在其中记录和保存您的日记:
HTML:
```html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>My Diary</title>
<link rel="stylesheet" href="style.css">
</head>
<body>
<header>
<h1>My Diary</h1>
</header>
<main>
<form>
<label for="title">Title:</label>
<input type="text" id="title" name="title" required>
<br>
<label for="date">Date:</label>
<input type="date" id="date" name="date" required>
<br>
<label for="content">Content:</label>
<textarea id="content" name="content" rows="10" required></textarea>
<br>
<button type="button" onclick="saveDiary()">Save</button>
</form>
<div id="diaryList"></div>
</main>
<script src="script.js"></script>
</body>
</html>
```
CSS:
```css
body {
margin: 0;
padding: 0;
font-family: Arial, sans-serif;
}
header {
background-color: #333;
color: white;
padding: 20px;
}
h1 {
margin: 0;
}
main {
padding: 20px;
}
form {
display: flex;
flex-direction: column;
max-width: 600px;
margin-bottom: 20px;
}
label {
margin-bottom: 5px;
}
input, textarea {
margin-bottom: 10px;
padding: 5px;
border: none;
border-radius: 5px;
box-shadow: 0 0 5px lightgray;
}
button {
padding: 5px 10px;
border: none;
border-radius: 5px;
background-color: #333;
color: white;
cursor: pointer;
}
button:hover {
background-color: #444;
}
```
JavaScript:
```javascript
// 获取当前日期
function getCurrentDate() {
const date = new Date();
const year = date.getFullYear();
const month = date.getMonth() + 1;
const day = date.getDate();
return `${year}-${month.toString().padStart(2, '0')}-${day.toString().padStart(2, '0')}`;
}
// 保存日记
function saveDiary() {
const title = document.getElementById('title').value;
const date = document.getElementById('date').value;
const content = document.getElementById('content').value;
const fileName = `${date} ${title}.txt`;
const fileContent = `Title: ${title}\nDate: ${date}\n\n${content}`;
const file = new Blob([fileContent], { type: 'text/plain;charset=utf-8' });
saveAs(file, fileName); // 使用FileSaver库保存文件
addDiaryToList(title, date, content); // 将日记添加到列表中
document.getElementById('title').value = '';
document.getElementById('date').value = getCurrentDate();
document.getElementById('content').value = '';
}
// 将日记添加到列表中
function addDiaryToList(title, date, content) {
const listItem = document.createElement('div');
listItem.className = 'diaryListItem';
listItem.innerHTML = `
<h3>${title}</h3>
<p>${date}</p>
<p>${content}</p>
`;
document.getElementById('diaryList').prepend(listItem);
}
// 初始化
document.getElementById('date').value = getCurrentDate(); // 设置默认日期
document.getElementById('title').focus(); // 自动聚焦到标题输入框
```
说明:
1. 该日记网站使用了FileSaver库,您需要将该库的JS文件下载并放置在与HTML文件相同的文件夹中。
2. 用户在网站上输入日记的标题、日期和内容后,点击“Save”按钮,网站将自动生成一个以日期和标题命名的txt文件,并将该文件保存在用户的本地计算机上。同时,该日记也会自动添加到日记列表中,供用户查看。
3. 该日记网站的样式较为简单,您可以根据需要进行修改和优化。
相关推荐













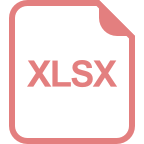