springboot 文件上传功能代码
时间: 2023-10-24 14:26:04 浏览: 48
下面是一个简单的 Spring Boot 文件上传示例代码:
1. 创建上传文件控制器
```java
@RestController
public class FileUploadController {
@PostMapping("/upload")
public String uploadFile(@RequestParam("file") MultipartFile file) {
if (file.isEmpty()) {
return "请选择要上传的文件";
}
try {
// 获取文件名
String fileName = file.getOriginalFilename();
// 获取文件内容
byte[] bytes = file.getBytes();
// 文件存储路径
String filePath = "/tmp/" + fileName;
// 创建文件
File dest = new File(filePath);
// 写入文件
FileUtils.writeByteArrayToFile(dest, bytes);
return "上传成功";
} catch (IOException e) {
e.printStackTrace();
return "上传失败";
}
}
}
```
2. 添加依赖
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
<version>2.5.3</version>
</dependency>
```
3. 配置文件上传大小限制
在 `application.properties` 或 `application.yml` 文件中添加以下配置:
```properties
# 限制文件上传大小为10MB
spring.servlet.multipart.max-file-size=10MB
spring.servlet.multipart.max-request-size=10MB
```
4. 创建上传文件的 HTML 页面
```html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>File Upload Example</title>
</head>
<body>
<h1>File Upload Example</h1>
<form method="POST" enctype="multipart/form-data" action="/upload">
<input type="file" name="file">
<br/><br/>
<input type="submit" value="Upload">
</form>
</body>
</html>
```
以上就是一个简单的 Spring Boot 文件上传示例,可以根据需求进行修改和扩展。
相关推荐
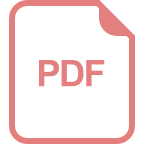
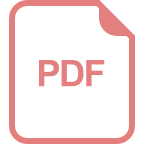
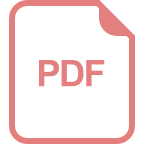
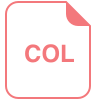
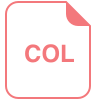
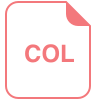
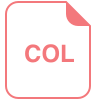
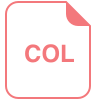









