matlab 椭圆检测
时间: 2023-06-20 10:08:50 浏览: 164
在Matlab中,可以使用图像处理工具箱中的函数来实现椭圆检测。下面简单介绍一下椭圆检测的步骤:
1. 读取待检测的图像
```
img = imread('test.jpg');
```
2. 转换为灰度图像并进行高斯滤波
```
gray_img = rgb2gray(img);
blur_img = imgaussfilt(gray_img, 2); % 根据实际情况选择合适的高斯核大小
```
3. 进行边缘检测
```
edge_img = edge(blur_img, 'canny');
```
4. 提取边缘上的点
```
[y, x] = find(edge_img);
```
5. 使用RANSAC算法拟合椭圆
```
% 定义拟合椭圆的函数
fit_ellipse = @(x,y) fit_ellipse_RANSAC(x, y, 0.01);
% 进行椭圆拟合
[model, inliers] = ransac([x y], fit_ellipse, 5, 1000);
```
6. 绘制拟合结果
```
imshow(img);
hold on;
plot(x(inliers), y(inliers), 'g.');
ellipse(model.a, model.b, model.phi, model.X0_in, model.Y0_in, 'r');
hold off;
```
其中,`fit_ellipse_RANSAC`函数是自己编写的用于拟合椭圆的函数,可以参考以下代码实现:
```
function [a, b, phi, X0, Y0] = fit_ellipse_RANSAC(x, y, t)
% RANSAC算法拟合椭圆
% x, y: 边缘点的坐标
% t: 拟合误差阈值
% 定义椭圆拟合函数
fit_ellipse = @(x) ellipse_error(x, [x(1) x(2) x(3) x(4) x(5)]);
% RANSAC参数
k = 5; % 最小样本数
N = 1000; % 迭代次数
P = 0.99; % 置信度
n = length(x); % 样本总数
% 初始化参数
a = 0;
b = 0;
phi = 0;
X0 = 0;
Y0 = 0;
best_inliers = [];
for i = 1:N
% 随机选择最小样本数个点
idx = randperm(n, k);
sample = [x(idx) y(idx)];
% 拟合椭圆
[model, ~] = fminsearch(fit_ellipse, [mean(sample(:,1)), mean(sample(:,2)), std(sample(:,1)), std(sample(:,2)), 0]);
% 计算误差
err = ellipse_error([x y], model);
% 判断是否为内点
inliers = find(err <= t);
% 判断是否为最优模型
if length(inliers) > length(best_inliers)
best_inliers = inliers;
a = model(3);
b = model(4);
phi = model(5);
X0 = model(1);
Y0 = model(2);
% 更新迭代次数
N = log(1 - P) / log(1 - (length(best_inliers) / n) ^ k);
end
end
end
function err = ellipse_error(xy, model)
% 计算椭圆拟合误差
% xy: 边缘点的坐标
% model: 椭圆参数
xc = model(1);
yc = model(2);
a = model(3);
b = model(4);
phi = model(5);
% 坐标旋转
xp = (xy(:,1) - xc) * cos(phi) + (xy(:,2) - yc) * sin(phi);
yp = (xy(:,2) - yc) * cos(phi) - (xy(:,1) - xc) * sin(phi);
% 计算误差
err = ((xp / a) .^ 2 + (yp / b) .^ 2 - 1) .^ 2;
end
```
以上就是使用Matlab进行椭圆检测的基本步骤,根据具体情况可以调整参数和算法来提高检测效果。
阅读全文
相关推荐
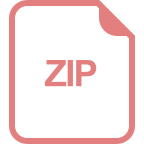
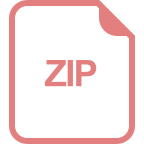
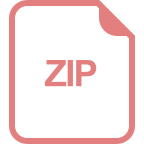
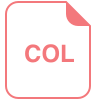
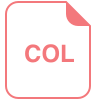
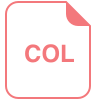
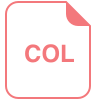
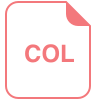
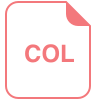
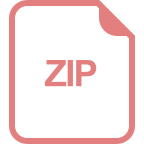

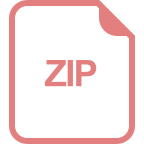

