koa框架中如何使用注解
时间: 2023-11-29 14:07:13 浏览: 34
在 Koa 框架中使用注解需要借助于 TypeScript,因为注解本身就是 TypeScript 中的一种特性。具体步骤如下:
1. 安装依赖:
```bash
npm install reflect-metadata
npm install @koa/router
npm install koa-bodyparser
npm install typescript
npm install ts-node
```
2. 在 tsconfig.json 中启用 decorator 和 emitDecoratorMetadata:
```json
{
"compilerOptions": {
"target": "es6",
"module": "commonjs",
"esModuleInterop": true,
"experimentalDecorators": true,
"emitDecoratorMetadata": true
}
}
```
3. 创建一个 Koa 应用,并且使用 koa-router 和 koa-bodyparser:
```typescript
import Koa from 'koa';
import Router from '@koa/router';
import bodyParser from 'koa-bodyparser';
const app = new Koa();
const router = new Router();
app.use(bodyParser());
app.use(router.routes());
```
4. 创建一个控制器类,使用 @Controller 装饰器标记该类,并使用 @Route 装饰器标记该类中的方法对应的路由:
```typescript
import { Controller, Route } from './decorators';
@Controller('/api')
class ApiController {
@Route('get', '/hello')
async sayHello(ctx: Koa.Context) {
ctx.body = 'Hello, world!';
}
}
```
5. 创建一个装饰器文件 decorators.ts,包含 @Controller 和 @Route 装饰器:
```typescript
import 'reflect-metadata';
import Router from '@koa/router';
const CONTROLLER_KEY = Symbol('controller');
const ROUTES_KEY = Symbol('routes');
export function Controller(path: string) {
return function(target: any) {
Reflect.defineMetadata(CONTROLLER_KEY, path, target);
}
}
export function Route(method: string, path: string) {
return function(target: any, key: string) {
if (!Reflect.hasMetadata(ROUTES_KEY, target.constructor)) {
Reflect.defineMetadata(ROUTES_KEY, [], target.constructor);
}
const routes = Reflect.getMetadata(ROUTES_KEY, target.constructor);
routes.push({ method, path, key });
}
}
export function registerRoutes(router: Router) {
const controllers = Reflect.getMetadata(CONTROLLER_KEY, Reflect) || [];
controllers.forEach((controller: any) => {
const routes = Reflect.getMetadata(ROUTES_KEY, controller) || [];
routes.forEach((route: any) => {
router[route.method](controller.path + route.path, controller[route.key]);
});
});
}
```
6. 在入口文件中注册路由:
```typescript
import { registerRoutes } from './decorators';
registerRoutes(router);
app.listen(3000);
```
以上就是在 Koa 框架中使用注解的基本步骤。
相关推荐
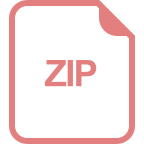
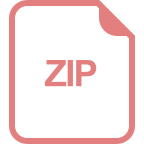
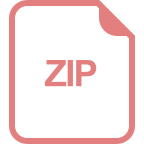
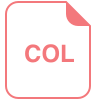
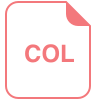












