(Rename files) Suppose you have a lot of files in a directory named Exercisei_j, where i and j are digits. Write a program that pads a 0 before i if i is a single digit. For example, a file named Exercise2_1 in a directory will be renamed to Exercise02_1. In Java, when you pass the symbol * from the command line, it refers to all files in the directory (see Supplement III.V). Use the following command to run your program: java Exercise12_28 *
时间: 2024-03-22 17:38:07 浏览: 29
Sure, I can help you with that! Here's the Java code to rename the files in the directory:
```java
import java.io.File;
public class Exercise12_28 {
public static void main(String[] args) {
String directoryPath = ".";
if (args.length > 0) {
directoryPath = args[0];
}
File directory = new File(directoryPath);
if (!directory.isDirectory()) {
System.err.println(directoryPath + " is not a directory");
return;
}
File[] files = directory.listFiles();
for (File file : files) {
String name = file.getName();
if (name.matches("Exercise\\d_\\d.*")) {
String[] parts = name.split("_");
String newName = "Exercise" + String.format("%02d", Integer.parseInt(parts[0].substring(8))) + "_" + parts[1];
File newFile = new File(directory, newName);
if (file.renameTo(newFile)) {
System.out.println(name + " renamed to " + newName);
} else {
System.err.println("Failed to rename " + name);
}
}
}
}
}
```
To run this program, you can compile it with `javac Exercise12_28.java` and then run it with `java Exercise12_28 *` to rename all files in the current directory. You can also specify a different directory path as the command-line argument.
This program uses regular expressions to match file names that start with "Exercise" followed by a single digit, an underscore, and another digit, and then renames those files by padding the first digit with a 0 if necessary. It also handles errors if the file cannot be renamed.
相关推荐
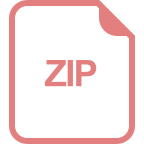
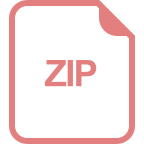
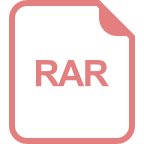










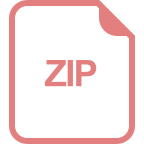
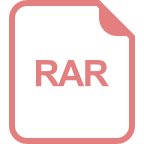
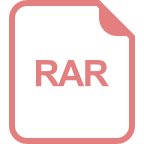