springboot的controller怎么接受前端传来的数组
时间: 2024-05-14 08:13:39 浏览: 119
您可以在Controller的方法参数中使用@RequestBody注解和List类型来接受前端传来的数组。例如:
```
@PostMapping("/array")
public void handleArray(@RequestBody List<String> array) {
// 处理数组
}
```
相关问题
springboot接收vue传来的数组
在Spring Boot中,可以使用@RequestBody注解来接收Vue传来的数组。首先,创建一个POJO类来表示数组的结构:
```java
public class MyArray {
private List<String> items;
public List<String> getItems() {
return items;
}
public void setItems(List<String> items) {
this.items = items;
}
}
```
然后,在Controller中使用@RequestBody注解将Vue传来的JSON数组转换为MyArray对象:
```java
@RestController
@RequestMapping("/api")
public class MyController {
@PostMapping("/myarray")
public void handleMyArray(@RequestBody MyArray myArray) {
List<String> items = myArray.getItems();
// 处理数组逻辑
}
}
```
在Vue中,可以使用axios库将数组传给Spring Boot:
```javascript
axios.post('/api/myarray', {
items: ['item1', 'item2', 'item3']
}).then(response => {
console.log(response.data);
}).catch(error => {
console.log(error);
});
```
在这个例子中,Vue将一个包含三个字符串元素的数组传给Spring Boot的/myarray接口,Spring Boot将这个数组转换为MyArray对象,然后可以在Controller中处理这个数组的逻辑。
springboot接收json数组
Spring Boot可以通过使用@RequestBody注解来接收JSON数组。具体步骤如下:
1. 在Controller中定义一个方法,使用@RequestBody注解来接收JSON数组。
```java
@PostMapping("/users")
public void createUsers(@RequestBody List<User> users) {
// 处理接收到的JSON数组
}
```
2. 定义一个User类,用于表示JSON数组中的每个元素。
```java
public class User {
private String name;
private int age;
// 省略getter和setter方法
}
```
3. 发送JSON数组到Controller的接口。
```json
[
{
"name": "张三",
"age": 20
},
{
"name": "李四",
"age": 25
}
]
```
以上就是使用Spring Boot接收JSON数组的步骤。
阅读全文
相关推荐
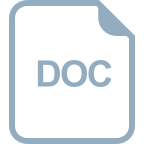
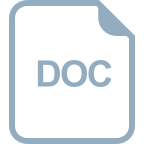
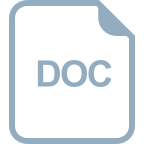
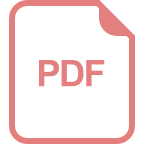
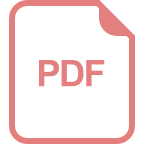
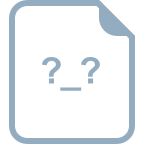
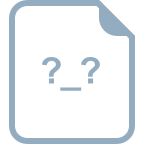
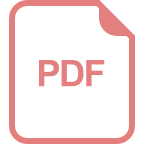
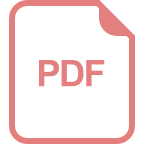
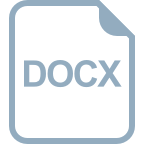
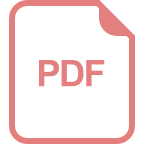
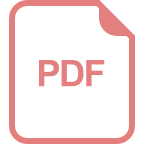
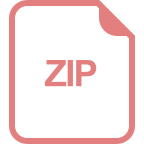
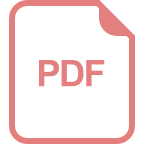