Qt的messagebox如何居中显示
时间: 2024-06-12 11:07:37 浏览: 216
可以使用以下代码:
```python
msgBox = QtWidgets.QMessageBox()
msgBox.setWindowTitle("Title")
msgBox.setText("Message")
msgBox.setStyleSheet("QMessageBox{qproperty-DQMessageBoxButtonLayoutDirection: Qt::RightToLeft;}")
msgBox.setWindowFlags(QtCore.Qt.WindowStaysOnTopHint | QtCore.Qt.FramelessWindowHint)
msgBox.move(QtGui.QGuiApplication.primaryScreen().geometry().center() - msgBox.rect().center())
msgBox.exec_()
```
其中,`setStyleSheet`是为了将按钮布局方向设置为从右往左,`setWindowFlags`是为了设置窗口置顶和无边框,`move`是为了将窗口移动到屏幕中央。
相关问题
如何使MessageBox居中显示?
要使MessageBox居中显示,可以使用以下步骤:
1. 获取屏幕的宽度和高度。
2. 获取MessageBox的宽度和高度。
3. 计算MessageBox的左上角坐标,使其位于屏幕中心。
4. 调用MessageBox函数,并将计算得到的坐标作为参数传入。
下面是一个示例代码,演示如何在C++中实现MessageBox居中显示:
```cpp
#include <windows.h>
void ShowCenteredMessageBox(HWND hwnd, LPCWSTR message, LPCWSTR title, UINT type)
{
// 获取屏幕的宽度和高度
int screenWidth = GetSystemMetrics(SM_CXSCREEN);
int screenHeight = GetSystemMetrics(SM_CYSCREEN);
// 获取MessageBox的宽度和高度
RECT rect;
MessageBox(hwnd, message, title, type | MB_SETFOREGROUND);
GetWindowRect(GetForegroundWindow(), &rect);
int messageBoxWidth = rect.right - rect.left;
int messageBoxHeight = rect.bottom - rect.top;
// 计算MessageBox的左上角坐标,使其位于屏幕中心
int x = (screenWidth - messageBoxWidth) / 2;
int y = (screenHeight - messageBoxHeight) / 2;
// 调用MessageBox函数,并将计算得到的坐标作为参数传入
MessageBox(hwnd, message, title, type | MB_SETFOREGROUND);
SetWindowPos(GetForegroundWindow(), HWND_TOPMOST, x, y, 0, 0, SWP_NOSIZE);
}
```
调用示例:
```cpp
int main()
{
HWND hwnd = NULL; // 窗口句柄,可以根据实际情况进行设置
LPCWSTR message = L"这是一条居中显示的消息";
LPCWSTR title = L"提示";
UINT type = MB_OK;
ShowCenteredMessageBox(hwnd, message, title, type);
return 0;
}
```
qt messagebox 显示指针
如果你想在Qt中使用QMessageBox来显示指针,可以使用QString的arg()函数将指针转换为字符串,并将其插入到消息框的文本中。
例如,以下示例代码在消息框中显示指针地址:
```cpp
void MyClass::showPointer(void* ptr)
{
QString message = QString("The pointer is: %1").arg((quintptr)ptr);
QMessageBox::information(this, "Pointer", message);
}
```
在上述代码中,quintptr是一个无符号整数类型,可以容纳指针的值。使用类型转换将指针转换为quintptr类型,并将其作为参数传递给arg()函数。arg()函数将quintptr类型转换为字符串,并将其插入到消息框的文本中。
阅读全文
相关推荐
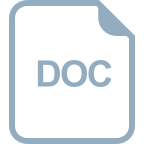
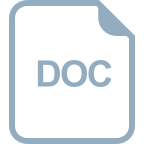
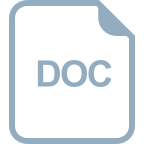
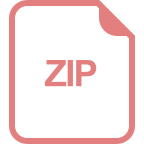
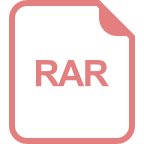






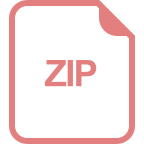
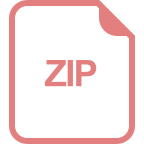
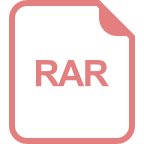
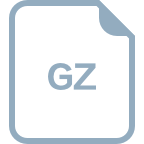
