使用html5做一个轮播图 可以左右滑动
时间: 2024-03-09 17:47:38 浏览: 32
可以使用HTML5和CSS3实现一个基本的轮播图。
HTML结构:
```html
<div class="carousel">
<div class="carousel-images">
<img src="image1.jpg">
<img src="image2.jpg">
<img src="image3.jpg">
</div>
</div>
```
CSS样式:
```css
.carousel {
width: 100%;
overflow: hidden;
}
.carousel-images {
display: flex;
width: 300%;
transition: transform 0.3s;
}
.carousel-images img {
width: 33.33%;
}
```
JS交互:
```js
var carouselImages = document.querySelector('.carousel-images');
var imageWidth = document.querySelector('.carousel-images img').clientWidth;
var currentImageIndex = 0;
function showNextImage() {
if (currentImageIndex < 2) {
currentImageIndex++;
carouselImages.style.transform = 'translateX(-' + (currentImageIndex * imageWidth) + 'px)';
}
}
function showPreviousImage() {
if (currentImageIndex > 0) {
currentImageIndex--;
carouselImages.style.transform = 'translateX(-' + (currentImageIndex * imageWidth) + 'px)';
}
}
setInterval(showNextImage, 3000);
```
解释:
1. `overflow: hidden`属性可以隐藏超出轮播图容器的图片部分。
2. `display: flex`和`width: 300%`属性可以将图片横向排列。
3. `transition: transform 0.3s`属性可以使图片在左右滑动时带有过渡效果。
4. `translateX(-' + (currentImageIndex * imageWidth) + 'px)'`属性可以实现图片的左右滑动,`currentImageIndex`表示当前显示的图片索引,`imageWidth`表示每张图片的宽度。
5. `setInterval`函数可以定时调用`showNextImage`函数,实现自动轮播。
完整代码如下:
```html
<!DOCTYPE html>
<html>
<head>
<title>轮播图</title>
<style>
.carousel {
width: 100%;
overflow: hidden;
}
.carousel-images {
display: flex;
width: 300%;
transition: transform 0.3s;
}
.carousel-images img {
width: 33.33%;
}
</style>
</head>
<body>
<div class="carousel">
<div class="carousel-images">
<img src="image1.jpg">
<img src="image2.jpg">
<img src="image3.jpg">
</div>
</div>
<script>
var carouselImages = document.querySelector('.carousel-images');
var imageWidth = document.querySelector('.carousel-images img').clientWidth;
var currentImageIndex = 0;
function showNextImage() {
if (currentImageIndex < 2) {
currentImageIndex++;
carouselImages.style.transform = 'translateX(-' + (currentImageIndex * imageWidth) + 'px)';
}
}
function showPreviousImage() {
if (currentImageIndex > 0) {
currentImageIndex--;
carouselImages.style.transform = 'translateX(-' + (currentImageIndex * imageWidth) + 'px)';
}
}
setInterval(showNextImage, 3000);
</script>
</body>
</html>
```
这样,就可以实现一个简单的左右滑动的轮播图了。如果需要添加小圆点和左右键功能,可以在HTML和JS中添加相关代码来实现。
相关推荐











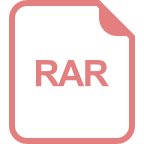
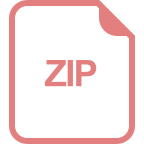