现在有一个图片,图片中有一个物体,现在已知物体外轮廓点坐标集,坐标系为图像坐标系,将图片绕图片中心旋转a°后,试求旋转后物体的外轮廓坐标点,依然用图像坐标系表示用python解决
时间: 2023-11-25 12:07:47 浏览: 32
可以使用旋转矩阵来实现物体的旋转。假设旋转中心为图片中心 $(x_c, y_c)$,旋转角度为 $a$。对于一个点 $(x_i, y_i)$,其旋转后的坐标为:
$$
\begin{pmatrix}
x_i' \\
y_i'
\end{pmatrix} =
\begin{pmatrix}
\cos a & -\sin a \\
\sin a & \cos a
\end{pmatrix}
\begin{pmatrix}
x_i - x_c \\
y_i - y_c
\end{pmatrix} +
\begin{pmatrix}
x_c \\
y_c
\end{pmatrix}
$$
实现代码如下:
``` python
import numpy as np
def rotate_points(points, center, angle):
"""在图像坐标系下旋转点集"""
# 将角度转换为弧度
angle_rad = np.deg2rad(angle)
# 计算旋转矩阵
c, s = np.cos(angle_rad), np.sin(angle_rad)
rotation_matrix = np.array([[c, -s], [s, c]])
# 将点集平移到原点
points_centered = points - center
# 旋转点集
points_rotated = np.dot(rotation_matrix, points_centered.T).T
# 将点集平移回原来位置
points_rotated += center
return points_rotated.astype(int) # 四舍五入取整,转为整数类型
```
其中,`points` 是形如 `(n, 2)` 的点集,`center` 是图片中心的坐标,`angle` 是旋转角度。函数返回旋转后的点集。
以下是一个示例:
``` python
import cv2
# 读取图片
img = cv2.imread('example.png')
# 计算图片中心
h, w = img.shape[:2]
center = np.array([w//2, h//2])
# 假设已知物体外轮廓点坐标集
points = np.array([[100, 100], [200, 100], [200, 200], [100, 200]])
# 将点集绘制在图片上
img_with_points = img.copy()
cv2.polylines(img_with_points, [points], True, (0, 0, 255), 2)
cv2.imshow('original', img_with_points)
# 对点集进行旋转
points_rotated = rotate_points(points, center, 30)
# 将旋转后的点集绘制在图片上
img_with_points_rotated = img.copy()
cv2.polylines(img_with_points_rotated, [points_rotated], True, (0, 0, 255), 2)
cv2.imshow('rotated', img_with_points_rotated)
cv2.waitKey(0)
```
其中,`example.png` 是一个示例图片,如下图所示:

运行结果如下图所示:

相关推荐
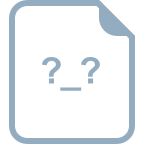
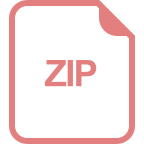














