数据结构c语言版插入
时间: 2023-06-30 21:12:44 浏览: 92
插入数据的常用数据结构有数组和链表,下面分别介绍它们的插入操作。
1. 数组插入
在数组中插入一个元素,需要将插入位置后面的元素依次后移,腾出插入位置。代码如下:
```c
void insert(int arr[], int size, int index, int value) {
if (index < 0 || index > size) {
printf("Invalid index!\n");
return;
}
// 将插入位置后面的元素后移
for (int i = size - 1; i >= index; i--) {
arr[i + 1] = arr[i];
}
// 插入元素
arr[index] = value;
printf("Insert %d at position %d.\n", value, index);
}
```
2. 链表插入
在链表中插入一个元素,需要找到插入位置的前一个节点,然后将新节点插入到该节点之后。代码如下:
```c
typedef struct node {
int data;
struct node *next;
} Node;
void insert(Node *head, int index, int value) {
if (index < 0) {
printf("Invalid index!\n");
return;
}
// 找到插入位置的前一个节点
Node *p = head;
for (int i = 0; i < index && p != NULL; i++) {
p = p->next;
}
if (p == NULL) {
printf("Invalid index!\n");
return;
}
// 插入新节点
Node *new_node = (Node *)malloc(sizeof(Node));
new_node->data = value;
new_node->next = p->next;
p->next = new_node;
printf("Insert %d at position %d.\n", value, index);
}
```
阅读全文
相关推荐
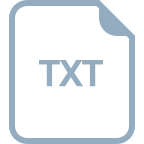
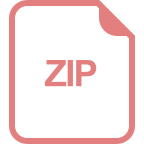
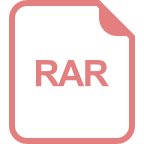
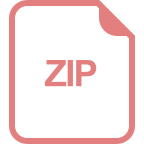
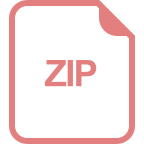
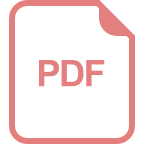
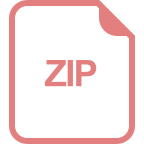
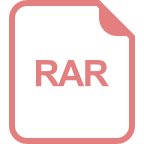
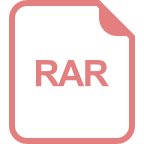
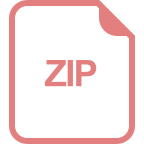
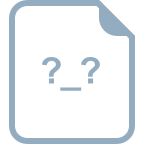
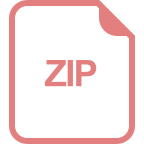
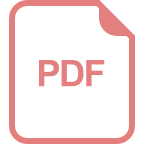