python把图片转换成pdf
时间: 2023-10-04 17:10:43 浏览: 57
可以使用Python的Pillow库和reportlab库来将图片转换成PDF。
首先需要安装这两个库,可以使用以下命令:
```
pip install Pillow
pip install reportlab
```
然后可以使用以下代码将图片转换成PDF:
```python
from PIL import Image
from reportlab.pdfgen import canvas
def image_to_pdf(image_path, pdf_path):
# 打开图片
image = Image.open(image_path)
# 获取图片的尺寸
width, height = image.size
# 创建一个新的PDF文件
pdf = canvas.Canvas(pdf_path, pagesize=(width, height))
# 将图片绘制到PDF文件中
pdf.drawImage(image_path, 0, 0)
# 保存PDF文件
pdf.save()
# 将图片转换成PDF
image_to_pdf('image.png', 'image.pdf')
```
在这个代码中,首先使用Pillow库打开图片,然后使用reportlab库创建一个新的PDF文件,将图片绘制到PDF文件中,最后保存PDF文件。需要将`image.png`替换成实际的图片路径,将`image.pdf`替换成想要保存的PDF文件路径。
相关问题
python如何将pdf转换成图片
### 回答1:
Python是一种广泛使用的编程语言,可以通过安装相关的第三方库来实现将PDF文件转换为图片。
首先,您需要安装Python的pdf2image库。该库可以将PDF文件转换为PIL图像,也就是Python Imaging Library图像。
安装pdf2image库后,您可以使用以下代码来将PDF文件转换为图像:
```python
from pdf2image import convert_from_path, convert_from_bytes
pages = convert_from_path('example.pdf', dpi=200)
for page in pages:
page.save('example.jpg', 'JPEG')
```
在上述代码中,convert_from_path函数将PDF文件的路径作为参数,并将dpi设置为200。这将生成一个包含所有PDF页面的列表。然后循环列表中的每一页,并将它们保存为JPEG文件。
您也可以将convert_from_path函数更改为convert_from_bytes函数,该函数可以读取二进制数据而不是文件名,并且可以使用options参数来更改图像的大小和质量。
总之,Python是一种非常强大和灵活的编程语言,可以用来处理几乎所有的任务,包括将PDF文件转换为图像。通过安装pdf2image库并调用相应的函数,您可以快速而轻松地将PDF文件转换为图像。
### 回答2:
Python可以使用一个强大的库来实现将PDF转换成图片的功能,这个库叫做“wand”。Wand库是用于在Python中进行ImageMagick的绑定,它可以与ImageMagick库无缝集成,提供了许多工具来处理图像,包括将PDF文件转换成图像的功能。
步骤如下所示:
1. 安装Wand库和ImageMagick库。你需要确保它们都被正确安装在你的计算机上。
2. 导入Wand库并加载所需的图像。你可以使用以下代码导入Wand库。
```
from wand.image import Image
```
然后你可以使用以下代码加载你的PDF文件。
```
with Image(filename='your_file.pdf', resolution=300) as img:
img.format = 'png'
img.save(filename='your_file.png')
```
在这个代码示例中,我们首先打开PDF文件,设置转换后的文件格式为PNG,并将转换后的文件保存在磁盘上。
3. 运行你的代码。运行上面的代码来将PDF文件转换成图像,并在你的磁盘上保存为PNG格式文件。你可以使用其他图像格式,比如JPEG,只需简单地使用“jpg”或“jpeg”代替“png”。
总之,使用Python将PDF文件转换成图像是非常简单的。使用Wand库,你可以轻松读取PDF文件并将其转换为图像,从而方便地在你的Python应用程序中使用它们。
### 回答3:
Python可以通过使用pdf2image库来将PDF文件转换成图片。首先需要安装pdf2image库,可以使用以下命令:
```
pip install pdf2image
```
接着,可以使用如下代码:
```python
from pdf2image import convert_from_path
# PDF文件路径
pdf_path = "example.pdf"
# 将PDF转换成图片
images = convert_from_path(pdf_path)
# 保存图片
for i, image in enumerate(images):
image.save(f"output_{i}.jpg", "JPEG")
```
上述代码中,我们使用pdf2image库中的`convert_from_path()`函数来将PDF转换成图片。该函数需要传入PDF文件路径,并返回一个图片列表。我们可以使用Python的循环语句将图片保存到指定的文件夹中。
需要注意的是,pdf2image库依赖于Poppler工具。如果在使用过程中遇到问题,可以尝试先安装Poppler工具,然后重新安装pdf2image库。
如何用python将图片转换为pdf
要将图片转换为 PDF,首先需要安装 Python 的 Pillow 库和 PyPDF2 库。
在命令行中输入以下命令来安装这两个库:
```
pip install Pillow
pip install PyPDF2
```
接下来就可以使用以下代码将图片转换为 PDF:
```python
from PIL import Image
import os
import PyPDF2
# 读取所有图片
imageList = []
for filename in os.listdir():
if filename.endswith(".jpg") or filename.endswith(".png"):
imagePath = os.path.abspath(filename)
imageList.append(imagePath)
# 对所有图片进行排序
imageList.sort()
# 创建PDF文档并添加所有图片
pdfWriter = PyPDF2.PdfFileWriter()
for imagePath in imageList:
im = Image.open(imagePath)
pdfWriter.addPage(im.convert('RGB'))
# 保存PDF文件
pdfOutput = open('output.pdf', 'wb')
pdfWriter.write(pdfOutput)
pdfOutput.close()
```
在运行代码之前,确保图片文件和代码文件在同一目录下,代码将读取该目录下的所有图片并将它们转换为一个名为“output.pdf”的 PDF 文件。
相关推荐
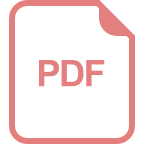
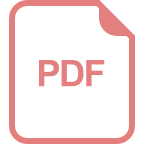











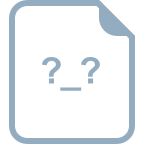
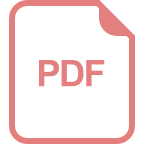