用java写一段CryptoJS解密的脚本
时间: 2023-08-27 10:15:44 浏览: 84
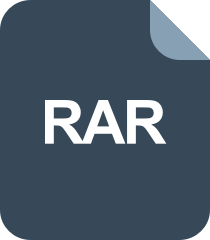
加密解密脚本编写

好的,以下是一个使用Java进行CryptoJS解密的示例代码:
```java
import java.util.Base64;
import javax.crypto.Cipher;
import javax.crypto.spec.IvParameterSpec;
import javax.crypto.spec.SecretKeySpec;
public class CryptoJSDemo {
public static void main(String[] args) throws Exception {
String key = "0123456789abcdef0123456789abcdef"; // 32 bytes key
String iv = "0123456789abcdef"; // 16 bytes iv
String encryptedText = "U2FsdGVkX18I8cEjE5dI2V4Pb3c7NkCtfk4W3lMF3hE="; // base64 encoded encrypted text
byte[] decodedKey = hexStringToByteArray(key);
byte[] decodedIV = hexStringToByteArray(iv);
byte[] decodedText = Base64.getDecoder().decode(encryptedText);
Cipher cipher = Cipher.getInstance("AES/CBC/PKCS5Padding");
SecretKeySpec secretKeySpec = new SecretKeySpec(decodedKey, "AES");
IvParameterSpec ivParameterSpec = new IvParameterSpec(decodedIV);
cipher.init(Cipher.DECRYPT_MODE, secretKeySpec, ivParameterSpec);
byte[] decryptedBytes = cipher.doFinal(decodedText);
String decryptedText = new String(decryptedBytes);
System.out.println(decryptedText);
}
public static byte[] hexStringToByteArray(String s) {
int len = s.length();
byte[] data = new byte[len / 2];
for (int i = 0; i < len; i += 2) {
data[i / 2] = (byte) ((Character.digit(s.charAt(i), 16) << 4)
+ Character.digit(s.charAt(i+1), 16));
}
return data;
}
}
```
请注意,此代码仅适用于使用AES算法和CBC模式进行加密的CryptoJS加密文本。您需要将key和iv替换为加密时使用的值,并将encryptedText替换为要解密的文本的base64编码版本。
阅读全文
相关推荐










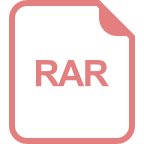
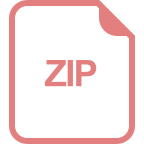



