winform openfiledialog excel
时间: 2023-07-21 19:25:00 浏览: 64
好的,你想要在 WinForm 中使用 OpenFileDialog 打开 Excel 文件,对吧?你可以按照以下步骤进行操作:
1. 首先,你需要在 WinForm 中添加 OpenFileDialog 控件。你可以在工具箱中找到 OpenFileDialog 控件,然后将它拖动到窗体中。
2. 接着,在你想要使用 OpenFileDialog 的按钮的 Click 事件中添加以下代码:
```
OpenFileDialog openFileDialog1 = new OpenFileDialog();
openFileDialog1.Filter = "Excel Files|*.xls;*.xlsx;*.xlsm";
if (openFileDialog1.ShowDialog() == DialogResult.OK)
{
string filePath = openFileDialog1.FileName;
// 进行Excel文件的读取操作
}
```
这段代码会打开一个 OpenFileDialog 窗口,允许用户选择 Excel 文件,并返回选择的文件路径。你可以在这个路径下进行 Excel 文件的读取操作。
3. 最后,你需要添加对 Microsoft.Office.Interop.Excel 的引用,以便在代码中访问 Excel 文件。你可以在 Visual Studio 中右键点击项目,选择“添加引用”,然后在“COM”选项卡中找到并勾选相应的 Microsoft Excel 库。
希望这些步骤能够对你有所帮助!
相关问题
winform读取excel
你可以通过以下步骤在 WinForm 中读取 Excel 文件:
1. 在 Visual Studio 中创建 WinForm 应用程序。
2. 在界面中添加一个 Button 控件和一个 DataGridView 控件。
3. 在 Button 的 Click 事件中添加以下代码:
```
private void button1_Click(object sender, EventArgs e)
{
// 打开文件对话框选择 Excel 文件
OpenFileDialog openFileDialog1 = new OpenFileDialog();
openFileDialog1.Filter = "Excel 文件|*.xls;*.xlsx";
if (openFileDialog1.ShowDialog() == DialogResult.OK)
{
// 获取 Excel 文件路径
string filePath = openFileDialog1.FileName;
// 连接 Excel 数据源
string connectionString = $"Provider=Microsoft.ACE.OLEDB.12.0;Data Source={filePath};Extended Properties='Excel 12.0;HDR=Yes;IMEX=1;'";
OleDbConnection connection = new OleDbConnection(connectionString);
connection.Open();
// 读取 Excel 数据
OleDbDataAdapter adapter = new OleDbDataAdapter("SELECT * FROM [Sheet1$]", connection);
DataTable dataTable = new DataTable();
adapter.Fill(dataTable);
// 将数据绑定到 DataGridView 控件
dataGridView1.DataSource = dataTable;
// 关闭连接
connection.Close();
}
}
```
这样,当用户点击 Button 控件时,就会打开文件对话框,让用户选择 Excel 文件,并将文件中的数据显示在 DataGridView 控件中。
winform读取excel并显示出来
在Winform中读取Excel并显示出来,可以通过使用第三方库来实现,比如EPPlus或NPOI。
首先,需要在Winform项目中引入相应的库。
然后,在Winform界面上添加一个"打开"按钮和一个数据表格控件DataGridView。
在打开按钮的点击事件中,打开Excel文件对话框,选择要读取的Excel文件。
接着,使用相应的库来读取Excel文件中的数据。以下以EPPlus为例:
```csharp
using OfficeOpenXml;
private void btnOpen_Click(object sender, EventArgs e)
{
// 打开Excel文件对话框
OpenFileDialog openFileDialog = new OpenFileDialog();
openFileDialog.Filter = "Excel Files|*.xls;*.xlsx;*.xlsm";
if (openFileDialog.ShowDialog() == DialogResult.OK)
{
// 获取选中的文件名
string fileName = openFileDialog.FileName;
// 读取Excel文件
ExcelPackage package = new ExcelPackage(new FileInfo(fileName));
// 获取第一个工作表
ExcelWorksheet worksheet = package.Workbook.Worksheets[1];
// 获取工作表中的数据
var data = worksheet.Cells.Value;
// 将数据显示在DataGridView中
dataGridView.DataSource = data;
}
}
```
以上代码中,通过ExcelPackage来打开Excel文件,然后获取第一个工作表,并将工作表中的数据赋值给DataGridView的DataSource属性,实现数据的显示。
需要注意的是,以上代码只是简单示例,实际应用中可能需要根据Excel文件的格式和结构进行相应的处理和逻辑。
相关推荐
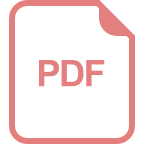
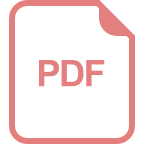












