JavaFX将项目中附件下载到本地
时间: 2024-02-01 08:12:13 浏览: 25
如果要在JavaFX应用程序中下载项目中的附件到本地,可以使用Java中的URLConnection类和JavaFX中的Task类来实现。
以下是一个简单的示例代码,可以从URL下载文件并将其保存到本地文件系统中:
```java
public class FileDownloader extends Task<Void> {
private String url;
private String localFilePath;
public FileDownloader(String url, String localFilePath) {
this.url = url;
this.localFilePath = localFilePath;
}
@Override
protected Void call() throws Exception {
URLConnection connection = new URL(url).openConnection();
try (InputStream in = connection.getInputStream();
FileOutputStream out = new FileOutputStream(localFilePath)) {
byte[] buffer = new byte[1024];
int bytesRead;
while ((bytesRead = in.read(buffer)) != -1) {
out.write(buffer, 0, bytesRead);
}
}
return null;
}
}
```
在JavaFX应用程序中,可以使用该类的实例来下载文件并使用JavaFX的ProgressBar来显示下载进度。例如:
```java
ProgressBar progressBar = new ProgressBar();
FileDownloader downloader = new FileDownloader("https://example.com/myfile.txt", "C:/temp/myfile.txt");
downloader.setOnSucceeded(event -> {
progressBar.setProgress(1.0);
});
downloader.setOnFailed(event -> {
progressBar.setProgress(0.0);
});
progressBar.progressProperty().bind(downloader.progressProperty());
new Thread(downloader).start();
```
这个示例中,将文件下载到了C:/temp/myfile.txt,如果需要下载其他类型的文件,只需要更改文件扩展名即可。
相关推荐
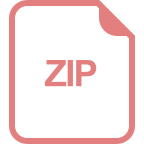














