用c语言写一个顺序建立链表的代码
时间: 2023-03-31 20:02:40 浏览: 118
可以的,以下是一个简单的顺序建立链表的 C 语言代码示例:
#include <stdio.h>
#include <stdlib.h>
struct Node {
int data;
struct Node* next;
};
int main() {
int n, i;
struct Node *head, *p, *q;
printf("请输入链表长度:");
scanf("%d", &n);
head = NULL;
for (i = 1; i <= n; i++) {
p = (struct Node*)malloc(sizeof(struct Node));
printf("请输入第 %d 个节点的值:", i);
scanf("%d", &p->data);
p->next = NULL;
if (head == NULL) {
head = p;
} else {
q->next = p;
}
q = p;
}
printf("链表的值为:");
p = head;
while (p != NULL) {
printf("%d ", p->data);
p = p->next;
}
printf("\n");
return ;
}
相关问题
c语言顺序建立链表代码
在C语言中,顺序创建链表通常是指手动分配内存并逐个添加节点的过程。这里是一个简单的单向链表的创建和插入节点的示例:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构体
typedef struct Node {
int data; // 节点数据
struct Node* next; // 指向下一个节点的指针
} Node;
// 创建一个新的链表节点
Node* createNode(int value) {
Node* newNode = (Node*)malloc(sizeof(Node)); // 分配内存
if (!newNode) {
printf("Memory allocation failed.\n");
return NULL;
}
newNode->data = value;
newNode->next = NULL; // 初始化新的节点为NULL,表示它是头结点
return newNode;
}
// 在链表末尾插入新节点
void insertAtEnd(Node** head, int value) {
Node* newNode = createNode(value); // 创建新节点
// 如果链表为空,则新节点既是头结点也是尾节点
if (*head == NULL) {
*head = newNode;
return;
}
// 遍历到链表末尾,并将新节点链接到最后一个元素之后
Node* current = *head;
while (current->next != NULL) {
current = current->next;
}
current->next = newNode;
}
// 打印链表
void printList(Node* head) {
Node* temp = head;
while (temp != NULL) {
printf("%d -> ", temp->data);
temp = temp->next;
}
printf("NULL\n");
}
int main() {
Node* head = NULL; // 初始化链表头部为NULL
// 插入一些节点
insertAtEnd(&head, 10);
insertAtEnd(&head, 20);
insertAtEnd(&head, 30);
// 打印链表
printList(head);
return 0;
}
```
在这个例子中,`createNode()`函数用于创建新的节点,`insertAtEnd()`函数负责在链表末尾插入节点,`printList()`则用于遍历并打印链表。
如何使用C语言实现一个功能:根据用户输入构建一个长度为5的链表,链表节点包含一个整数,并按照输入顺序建立链表,然后逐个打印链表中的节点数据?
在C语言中,我们可以使用结构体(struct)来表示链表节点,同时使用指针来构建链表。以下是一个简单的步骤示例:
1. 定义链表节点结构体:
```c
typedef struct Node {
int data; // 节点存储的整数值
struct Node* next; // 指向下一个节点的指针
} Node;
```
2. 创建链表并插入节点:
```c
Node* createList(int input[]) {
Node* head = NULL;
Node* current = NULL;
for (int i = 0; i < 5; i++) {
Node newNode;
newNode.data = input[i];
newNode.next = NULL;
if (!head) {
head = &newNode;
current = head;
} else {
current->next = &newNode;
current = current->next;
}
}
return head;
}
```
这里假设你已经有了一个包含5个整数的一维数组input[],这个函数会遍历数组并将元素插入链表中。
3. 打印链表节点:
```c
void printList(Node* head) {
Node* temp = head;
while (temp != NULL) {
printf("%d ", temp->data);
temp = temp->next;
}
printf("\n");
}
```
你可以先调用`createList`函数创建链表,然后调用`printList`函数来显示链表内容。
完整代码示例:
```c
#include <stdio.h>
// ... (上述链表节点和链表操作的定义)
int main() {
int input[] = {1, 2, 3, 4, 5};
Node* listHead = createList(input);
printList(listHead);
return 0;
}
```
运行这段代码,你会看到按顺序打印出的链表节点数据:1 2 3 4 5。
阅读全文
相关推荐
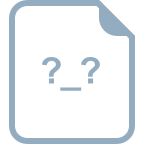
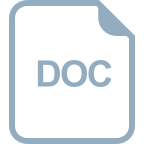




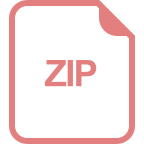
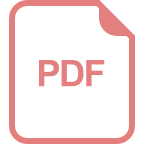
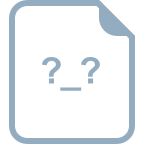
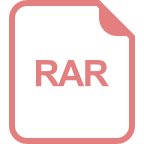
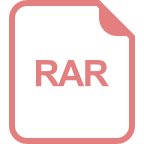



