bool OrderAction() { CThostFtdcInputOrderActionField t={0}; strcpy(t.BrokerID, "1000"); strcpy(t.ExchangeID, "SSE"); strcpy(t.OrderSysID, ) ; void OnRtnOrder(CThostFtdcOrderField *pOrder) { printf("OnRtnOrder\n"); std::cout << "OrderSysID: " << pOrder->OrderSysID << std::endl; } 如何在OrderAction()中的t.OrderSysID指向OnRtnOrder()中的OrderSysID
时间: 2023-12-06 12:45:39 浏览: 133
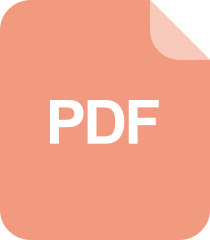
T-SQL中使用正则表达式函数
需要在OrderAction()函数中保存OrderSysID,然后在OnRtnOrder()函数中获取并使用它。可以通过定义一个全局变量来实现:
```
std::string g_orderSysID; // 全局变量保存OrderSysID
bool OrderAction() {
CThostFtdcInputOrderActionField t={0};
strcpy(t.BrokerID, "1000");
strcpy(t.ExchangeID, "SSE");
strcpy(t.OrderSysID, g_orderSysID.c_str()); // 将全局变量中保存的OrderSysID赋值给t.OrderSysID
// ... 其他代码
}
void OnRtnOrder(CThostFtdcOrderField *pOrder) {
printf("OnRtnOrder\n");
std::cout << "OrderSysID: " << pOrder->OrderSysID << std::endl;
g_orderSysID = pOrder->OrderSysID; // 将获取到的OrderSysID保存到全局变量中
// ... 其他代码
}
```
这样,在OrderAction()函数中就可以使用OnRtnOrder()函数中获取到的OrderSysID了。
阅读全文
相关推荐
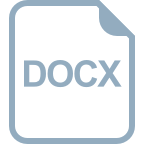
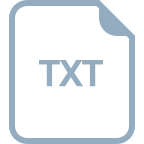

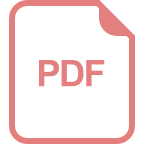
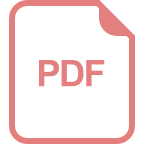
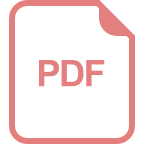
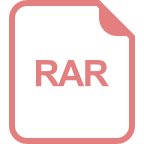
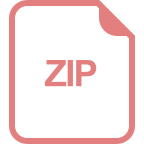
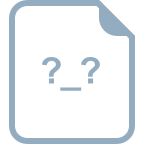
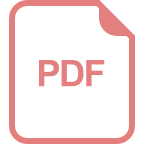
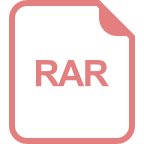
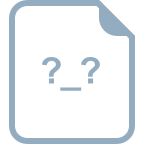
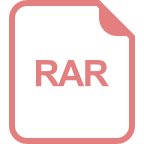