给一个列表和一个整数,删除列表中能被该数整除的列表元素
时间: 2024-04-03 10:10:40 浏览: 29
以下是Python的实现代码:
```python
def remove_divisible(lst, n):
return [x for x in lst if x % n != 0]
```
这个函数接收一个列表 `lst` 和一个整数 `n`,返回一个新的列表,其中不包含原列表中能被 `n` 整除的元素。
例如,对于列表 `[1, 2, 3, 4, 5, 6, 7, 8, 9]` 和整数 `3`,调用 `remove_divisible` 函数会返回 `[1, 2, 4, 5, 7, 8]`。
相关问题
Python给一个列表,和一个整数,删除列表中索引(从0开始)能被该数整除的列表元素
好的,这个问题可以回答。可以通过遍历列表,判断索引是否能被整数整除,然后删除对应的元素。具体的实现可以参考以下代码:
```
def remove_elements(lst, n):
idx = 0
while idx < len(lst):
if idx % n == 0:
lst.pop(idx)
else:
idx += 1
return lst
# example usage
my_list = ['a', 'b', 'c', 'd', 'e', 'f']
n = 3
result = remove_elements(my_list, n)
print(result) # Output: ['a', 'b', 'd', 'e']
```
这段代码中,`remove_elements` 函数接受一个列表和一个整数参数,然后对列表进行遍历和删除操作。具体来说,我们使用一个 `while` 循环和一个索引变量 `idx` 来遍历列表。在遍历的过程中,我们判断当前索引是否能被整数参数整除,如果是的话,就删除对应的元素,否则就将索引变量加 1,继续遍历下一个元素。最后,我们返回更新后的列表。
python给一个列表,和一个整数,删除列表中索引(从0开始)能被该数整除的列表元素
可以使用Python中的列表推导式来实现:
```python
new_list = [item for index, item in enumerate(old_list) if (index+1) % divisor != 0]
```
其中,`old_list`是原始列表,`divisor`是整数,`new_list`是删除索引为该整数倍数的元素后的新列表。
解释一下列表推导式的含义:对于`old_list`中的每个元素`item`和对应的索引`index`,只有当`(index+1) % divisor`不等于0时,才将该元素加入到`new_list`中。`(index+1)`用于将索引从从0开始变成从1开始,因为题目中要求从1开始计算索引。
相关推荐
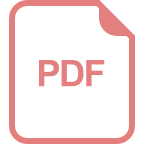












