C#如何实现缩圈坐标缩圈
时间: 2023-07-12 12:16:43 浏览: 40
缩圈坐标缩圈也是一种图像处理技术,可以缩小圆形区域的大小,同时缩小圆形区域中的坐标值。在C#中,可以使用相同的方法来实现缩圈坐标缩圈。
以下是示例代码:
```csharp
private PointF ShrinkPoint(PointF point, PointF center, float shrinkSize)
{
// 计算缩圈后的半径
float radius = (float)Math.Sqrt(Math.Pow(point.X - center.X, 2) + Math.Pow(point.Y - center.Y, 2));
float newRadius = radius - shrinkSize;
// 计算缩圈后的坐标
float angle = (float)Math.Atan2(point.Y - center.Y, point.X - center.X);
float newX = center.X + newRadius * (float)Math.Cos(angle);
float newY = center.Y + newRadius * (float)Math.Sin(angle);
return new PointF(newX, newY);
}
private void DrawShrinkCircle(Graphics g, int centerX, int centerY, int radius, int shrinkSize)
{
// 计算缩圈后的半径
int newRadius = radius - shrinkSize;
// 创建GraphicsPath对象
GraphicsPath path = new GraphicsPath();
path.AddEllipse(centerX - newRadius, centerY - newRadius, newRadius * 2, newRadius * 2);
path.AddEllipse(centerX - radius, centerY - radius, radius * 2, radius * 2);
// 创建PathGradientBrush对象
PathGradientBrush brush = new PathGradientBrush(path);
brush.CenterPoint = new PointF(centerX, centerY);
brush.CenterColor = Color.Transparent;
brush.SurroundColors = new Color[] { Color.Black };
// 绘制缩圈后的圆形区域
g.FillEllipse(brush, centerX - newRadius, centerY - newRadius, newRadius * 2, newRadius * 2);
}
```
这个示例代码中,我们首先定义了一个ShrinkPoint方法,用于计算缩圈后的坐标。然后,我们在DrawShrinkCircle方法中调用了这个方法,将缩圈后的坐标传递给GraphicsPath对象,用于创建缩圈后的圆形区域。
使用示例代码:
```csharp
private void pictureBox1_Paint(object sender, PaintEventArgs e)
{
// 绘制原始圆形区域
e.Graphics.DrawEllipse(Pens.Red, 50, 50, 100, 100);
// 绘制缩圈后的圆形区域
DrawShrinkCircle(e.Graphics, 100, 100, 50, 10);
// 绘制坐标点
PointF point = new PointF(75, 75);
PointF newPoint = ShrinkPoint(point, new PointF(100, 100), 10);
e.Graphics.FillEllipse(Brushes.Blue, newPoint.X - 3, newPoint.Y - 3, 6, 6);
}
```
在使用示例代码中,我们在一个PictureBox控件的Paint事件中绘制了原始圆形区域、缩圈后的圆形区域和一个坐标点。我们通过ShrinkPoint方法计算出了缩圈后的坐标点,并使用FillEllipse方法绘制了这个点。
相关推荐
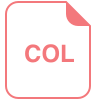
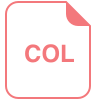
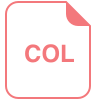
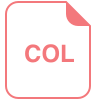
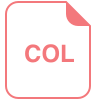









