The value of expression *((int *)(p+1)+2) is __. static struct { int x, y[3]; } a[3] = {{1,2,3,4},{5,6,7,8},{9,10,11,12}}, *p; p = a+1;
时间: 2023-10-06 17:07:25 浏览: 517
The value of expression `*((int *)(p+1)+2)` can be calculated as follows:
1. `p+1` points to the second element of the array `a`.
2. Casting `p+1` to an `int *` pointer type means that the pointer arithmetic will be performed based on the size of `int`, which is typically 4 bytes. So, `p+1` will be incremented by `sizeof(int)` bytes.
3. Adding 2 to the resulting pointer `(int *)(p+1)+2` means that it will be incremented by `2 * sizeof(int)` bytes.
4. Finally, dereferencing the resulting pointer `*((int *)(p+1)+2)` gives us the value of the third integer in the second element of the array `a`, which is `7`.
Therefore, the value of expression `*((int *)(p+1)+2)` is `7`.
相关问题
问题描述】简单的语法分析程序设计 【输入形式】随机输入赋值语句 【输出形式】相应的四元式序列 同时输出所输入的赋值语句与相应的四元式序列以作对照。 【要求】 1. 采用递归下降分析程序完成(自上而下的分析) 2. 确定各个子程序的功能并画出流程图 3.文法如下: [1525673712688015646.jpg] [1525673712700021141.jpg] 4. 编码、调试通过(C语言编写) 5. 设计3-5个赋值语句测试实例,检验程序能否输出正确的四元式;当输入错误的句子时,检验程序能够给出语法错误的相应提示信息。 【样例输入】 x:=a+b*c/d-(e+f) 【样例输出】 T1:=b*c (*,b,c,T1) T2:=T1/d (/,T1,d,T2) T3:=a+T2 (+,a,T2,T3) T4:=e+f (+,e,f,T4) T5:=T3-T4 (-,T3,T4,T5) x:=T5 (:=,T5,-,x) 【样例说明】 语句和四元式之间用5个空格隔开; 程序除能够正确输出四元式外,当输入的表达式错误时,还应能检测出语法错误,给出相应错误提示。
这是一个关于简单语法分析程序设计的问题,要求使用递归下降分析程序完成,并输出相应的四元式序列。同时,需要画出各个子程序的流程图,并且能够处理输入的错误语句并给出相应的提示信息。
该问题的文法如下:
```
<表达式> → <项>{<加法运算符><项>}
<项> → <因子>{<乘法运算符><因子>}
<因子> → <标识符>|<无符号整数>|‘(’<表达式>‘)’
<加法运算符> → +|-
<乘法运算符> → *|/
```
其中,`<标识符>`表示变量名,`<无符号整数>`表示非负整数。
下面是一个可能的解法:
```c
#include <stdio.h>
#include <stdlib.h>
#include <ctype.h>
#define MAXLEN 100
/* 定义四元式结构体 */
typedef struct {
char op; /* 操作符 */
char arg1[MAXLEN]; /* 第一个操作数 */
char arg2[MAXLEN]; /* 第二个操作数 */
char result[MAXLEN];/* 结果 */
} Quaternary;
/* 定义全局变量 */
char lookahead; /* 当前读入字符 */
char token[MAXLEN]; /* 当前读入的标识符或数字 */
char *expression; /* 表达式字符串指针 */
Quaternary q[MAXLEN]; /* 保存四元式的数组 */
int qcount = 0; /* 已生成的四元式数量 */
/* 前向声明 */
void expression();
void term();
void factor();
void error(char *msg);
/* 检查是否为运算符 */
int is_operator(char c) {
return c == '+' || c == '-' || c == '*' || c == '/';
}
/* 读入下一个字符 */
void next_char() {
lookahead = *expression++;
}
/* 跳过空格 */
void skip_white_space() {
while (isspace(lookahead)) {
next_char();
}
}
/* 读入标识符或数字 */
void read_token() {
int i = 0;
while (isalnum(lookahead)) {
token[i++] = lookahead;
next_char();
}
token[i] = '\0';
}
/* 读入一个整数 */
int read_integer() {
int value = 0;
while (isdigit(lookahead)) {
value = value * 10 + lookahead - '0';
next_char();
}
return value;
}
/* 生成四元式 */
void gen(char op, char *arg1, char *arg2, char *result) {
q[qcount].op = op;
strcpy(q[qcount].arg1, arg1);
strcpy(q[qcount].arg2, arg2);
strcpy(q[qcount].result, result);
qcount++;
}
/* 生成一个新的临时变量 */
char *new_temp() {
static int temp_num = 0;
char *temp = malloc(MAXLEN);
sprintf(temp, "T%d", temp_num++);
return temp;
}
/* 处理加法 */
void add() {
match('+');
term();
char *temp = new_temp();
gen('+', token, q[qcount-1].result, temp);
strcpy(q[qcount-1].result, temp);
free(temp);
}
/* 处理减法 */
void subtract() {
match('-');
term();
char *temp = new_temp();
gen('-', token, q[qcount-1].result, temp);
strcpy(q[qcount-1].result, temp);
free(temp);
}
/* 处理乘法 */
void multiply() {
match('*');
factor();
char *temp = new_temp();
gen('*', token, q[qcount-1].result, temp);
strcpy(q[qcount-1].result, temp);
free(temp);
}
/* 处理除法 */
void divide() {
match('/');
factor();
char *temp = new_temp();
gen('/', q[qcount-1].result, token, temp);
strcpy(q[qcount-1].result, temp);
free(temp);
}
/* 匹配一个字符 */
void match(char c) {
if (lookahead == c) {
next_char();
skip_white_space();
} else {
char msg[MAXLEN];
sprintf(msg, "Expected '%c', but got '%c'", c, lookahead);
error(msg);
}
}
/* 处理错误 */
void error(char *msg) {
printf("Error: %s\n", msg);
exit(1);
}
/* 处理表达式 */
void expression() {
term();
while (lookahead == '+' || lookahead == '-') {
if (lookahead == '+') {
add();
} else if (lookahead == '-') {
subtract();
}
}
}
/* 处理项 */
void term() {
factor();
while (lookahead == '*' || lookahead == '/') {
if (lookahead == '*') {
multiply();
} else if (lookahead == '/') {
divide();
}
}
}
/* 处理因子 */
void factor() {
if (isalpha(lookahead)) {
read_token();
if (lookahead == '=') { /* 处理赋值语句 */
match('=');
expression();
gen('=', q[qcount-1].result, "", token);
} else { /* 处理变量 */
gen(' ', token, "", token);
}
} else if (isdigit(lookahead)) {
int value = read_integer();
char int_str[MAXLEN];
sprintf(int_str, "%d", value);
gen(' ', int_str, "", int_str);
} else if (lookahead == '(') {
match('(');
expression();
match(')');
} else {
error("Expected identifier, integer, or '('");
}
}
int main() {
char input[MAXLEN];
printf("Enter an expression: ");
fgets(input, MAXLEN, stdin);
expression = input;
skip_white_space();
expression();
printf("Expression: %s\n", input);
printf("Quaternary sequence:\n");
for (int i = 0; i < qcount; i++) {
printf("%s %c %s %s\n", q[i].result, q[i].op, q[i].arg1, q[i].arg2);
}
return 0;
}
```
上面的代码中,我们定义了一个 `Quaternary` 结构体来保存每个四元式的信息,包括操作符、两个操作数和结果。同时,我们也定义了全局变量 `q` 来保存所有生成的四元式。在程序中,我们通过调用 `gen` 函数来生成新的四元式,并通过 `new_temp` 函数来生成一个新的临时变量名。
在 `expression`、`term` 和 `factor` 函数中,我们按照文法规则递归下降处理表达式,并在必要时生成相应的四元式。注意,在 `factor` 函数中,我们需要处理赋值语句和变量名这两种情况。
最后,我们在 `main` 函数中读入输入的表达式,并调用 `expression` 函数来处理它。在输出时,我们先输出原始表达式,然后逐行输出生成的四元式序列。
中缀表达式指二元运算符位于两个操作数中间,例如2+37。编写程序计算中缀表达式的结果值。 输入:一行内给出不超过30个字符的中缀表达式,只包含+-/及运算数,不同对象(运算数、运算符号)之间以空格分隔。 输出:中缀表达式的结果,精确到小数点后1位,或错误信息“ERROR”。
要编写一个C++程序来计算中缀表达式的结果,我们可以使用递归解析器的方法。首先,我们需要定义一个数据结构表示操作数和运算符,然后创建函数来处理中缀表达式的各个步骤。以下是一个简单的实现:
```cpp
#include <iostream>
#include <stack>
#include <cctype>
// 定义数据结构来存储操作数和运算符
struct ExpressionToken {
enum Type { NUMBER, PLUS, MINUS, DIVIDE, MULTIPLY };
union {
double value;
char op;
} data;
Type type;
};
// 解析函数,处理中缀表达式
double evaluateInfix(const std::string& infix) {
ExpressionToken tokens[30]; // 假设最大表达式长度为30
int tokenIndex = 0;
for (char c : infix) {
if (isdigit(c)) {
tokens[tokenIndex].data.value = static_cast<double>(c - '0');
tokens[tokenIndex].type = ExpressionToken::NUMBER;
} else if (c == '+' || c == '-' || c == '*' || c == '/') {
tokens[tokenIndex].data.op = c;
tokens[tokenIndex].type = static_cast<ExpressionToken::Type>(c);
++tokenIndex;
}
}
// 如果没有读取完整个表达式,填充剩余位置
while (tokenIndex < 30) {
tokens[tokenIndex].data.value = 0; // 默认值为0
tokens[tokenIndex].type = ExpressionToken::NUMBER;
++tokenIndex;
}
return evaluateTokens(tokens, tokenIndex);
}
// 递归计算表达式
double evaluateTokens(const ExpressionToken* tokens, int numTokens) {
if (numTokens <= 1) {
return tokens[0].data.value;
}
double left = evaluateTokens(tokens, tokens[numTokens - 2]);
double right = evaluateTokens(tokens + numTokens - 2, 2);
switch (tokens[numTokens - 1].type) {
case ExpressionToken::PLUS:
return left + right;
case ExpressionToken::MINUS:
return left - right;
case ExpressionToken::MULTIPLY:
return left * right;
case ExpressionToken::DIVIDE:
if (right != 0)
return left / right;
else
return "ERROR"; // 除数不能为零
default:
throw std::runtime_error("Invalid operator");
}
}
int main() {
std::string infixExpression;
std::cout << "Enter a valid infix expression (up to 30 characters): ";
std::getline(std::cin, infixExpression);
try {
double result = evaluateInfix(infixExpression);
std::cout << "Result: " << std::fixed << result << ".0" << std::endl;
} catch (const std::exception& e) {
std::cerr << "Error: " << e.what() << std::endl;
}
return 0;
}
```
在这个程序中,我们首先将输入的字符串分割成操作数和运算符,然后递归地计算它们之间的结果。如果遇到无效的运算符或者除数为零,我们会抛出异常并打印错误消息。
阅读全文
相关推荐
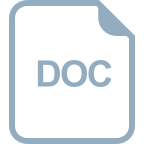
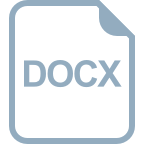
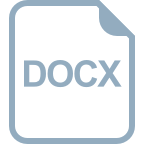
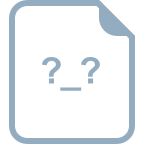
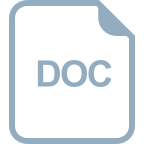
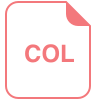
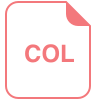
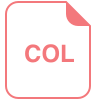
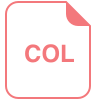
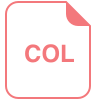
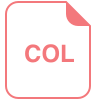
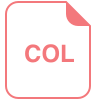
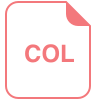
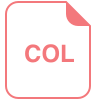
