php使用函数实现购物车加减,zencart购物车实现加减框
时间: 2024-01-06 14:07:13 浏览: 89
在 PHP 中,可以使用函数来实现购物车的加减功能。以下是一个基本的示例代码:
```
<?php
session_start();
// 添加商品到购物车
function addToCart($product_id, $quantity) {
// 如果购物车中已经有该商品,则增加数量
if (isset($_SESSION['cart'][$product_id])) {
$_SESSION['cart'][$product_id] += $quantity;
}
// 否则,将商品添加到购物车
else {
$_SESSION['cart'][$product_id] = $quantity;
}
}
// 从购物车中删除商品
function removeFromCart($product_id) {
if (isset($_SESSION['cart'][$product_id])) {
unset($_SESSION['cart'][$product_id]);
}
}
// 更新购物车中商品的数量
function updateCart($product_id, $quantity) {
if (isset($_SESSION['cart'][$product_id])) {
$_SESSION['cart'][$product_id] = $quantity;
}
}
// 获取购物车中商品的数量
function getCartQuantity($product_id) {
if (isset($_SESSION['cart'][$product_id])) {
return $_SESSION['cart'][$product_id];
} else {
return 0;
}
}
?>
```
在 ZenCart 中,可以使用以下代码来在购物车页面中实现加减框:
```
<input type="text" name="products_quantity[]" size="4" value="<?php echo $products_quantity; ?>">
<input type="button" value="-" onclick="javascript:update_quantity(-1, '<?php echo $products_id; ?>');">
<input type="button" value="+" onclick="javascript:update_quantity(1, '<?php echo $products_id; ?>');">
<script>
function update_quantity(change, product_id) {
var quantity_input = document.getElementsByName('products_quantity[' + product_id + ']')[0];
var quantity = parseInt(quantity_input.value) + change;
if (quantity < 1) {
quantity = 1;
}
quantity_input.value = quantity;
}
</script>
```
这段代码会在每个商品数量输入框旁边生成一个“-”和“+”按钮,点击“-”按钮会将商品数量减1,点击“+”按钮会将商品数量加1。需要注意的是,这段代码中的 `$products_id` 和 `$products_quantity` 变量需要根据具体情况进行替换。
阅读全文
相关推荐
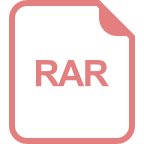
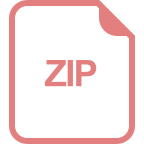
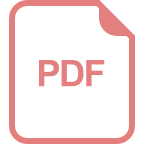



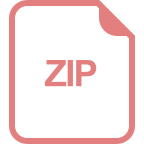
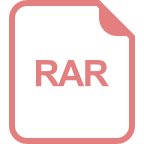
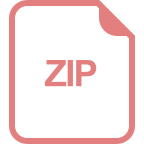
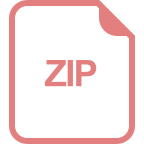
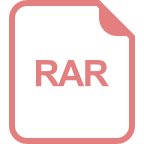
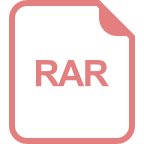
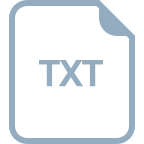
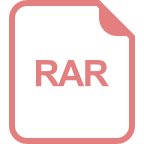
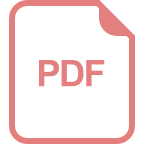
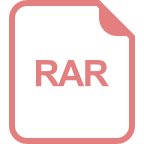
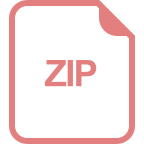
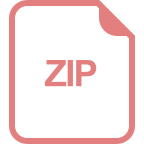