C++ UTF16BE编码的char数组转成UTF8,代码展示
时间: 2023-09-12 22:05:06 浏览: 51
下面是将UTF16BE编码的char数组转换成UTF8的示例代码:
```c++
#include <iostream>
#include <string>
using namespace std;
string utf16be_to_utf8(const char* utf16be, size_t len) {
string utf8;
uint32_t codepoint;
for (size_t i = 0; i < len; i += 2) {
codepoint = (utf16be[i] << 8) | utf16be[i+1];
if (codepoint <= 0x7F) {
utf8 += static_cast<char>(codepoint);
} else if (codepoint <= 0x7FF) {
utf8 += static_cast<char>(0xC0 | ((codepoint >> 6) & 0x1F));
utf8 += static_cast<char>(0x80 | (codepoint & 0x3F));
} else if (codepoint <= 0xFFFF) {
utf8 += static_cast<char>(0xE0 | ((codepoint >> 12) & 0x0F));
utf8 += static_cast<char>(0x80 | ((codepoint >> 6) & 0x3F));
utf8 += static_cast<char>(0x80 | (codepoint & 0x3F));
} else {
utf8 += static_cast<char>(0xF0 | ((codepoint >> 18) & 0x07));
utf8 += static_cast<char>(0x80 | ((codepoint >> 12) & 0x3F));
utf8 += static_cast<char>(0x80 | ((codepoint >> 6) & 0x3F));
utf8 += static_cast<char>(0x80 | (codepoint & 0x3F));
}
}
return utf8;
}
int main() {
// 示例输入为 "你好"
char utf16be[] = {0x4F, 0x60, 0x59, 0x6D}; // UTF16BE编码的char数组
size_t len = sizeof(utf16be) / sizeof(char);
string utf8 = utf16be_to_utf8(utf16be, len);
cout << "UTF8编码的字符串为: " << utf8 << endl;
return 0;
}
```
在上面的代码中,我们定义了一个 `utf16be_to_utf8` 函数,它的作用是将UTF16BE编码的char数组转换成UTF8编码的字符串。接着在 `main` 函数中,我们定义了一个UTF16BE编码的char数组 `utf16be`,并将其传递给 `utf16be_to_utf8` 函数进行转换。最后输出转换后的UTF8编码的字符串。
需要注意的是,UTF16BE编码中,每个字符占用两个字节,因此在 `utf16be_to_utf8` 函数中,我们每次读取两个字节,并将其转换成一个Unicode码点。在将Unicode码点转换成UTF8编码时,我们采用了UTF8的标准转换方式,即:
- 如果码点小于等于0x7F,则转换成一个字节的UTF8编码;
- 如果码点小于等于0x7FF,则转换成两个字节的UTF8编码;
- 如果码点小于等于0xFFFF,则转换成三个字节的UTF8编码;
- 如果码点小于等于0x10FFFF,则转换成四个字节的UTF8编码。
最后,我们将每个Unicode码点转换成的UTF8编码拼接成一个完整的UTF8编码的字符串。
相关推荐
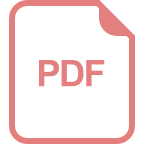
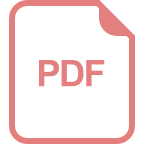
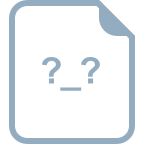














