Constructor c2 = clazz.getDeclaredConstructor(String.class,int.class)
时间: 2024-02-13 13:01:50 浏览: 73
这是Java中的一个方法,它可以获取类的指定构造方法。在这个例子中,`clazz`是一个Class对象,表示一个类,`getDeclaredConstructor`方法可以获取该类中声明的构造方法,参数`String.class`和`int.class`表示构造方法的参数类型列表。这里获取的是一个带有`String`和`int`两个参数的构造方法。
获取构造方法后,可以通过`newInstance`方法来创建对象,例如:
```java
Constructor c2 = clazz.getDeclaredConstructor(String.class,int.class);
Object obj = c2.newInstance("example", 123);
```
这样就可以创建一个带有`String`和`int`两个参数的对象。需要注意的是,如果要调用非public的构造方法,需要先调用`setAccessible(true)`方法来取消Java的访问控制检查。
相关问题
访问setName方法报错,怎么改正 Constructor constructor = clazz.getConstructor(); Object o = constructor.newInstance(); Method setName = clazz.getMethod("setName",clazz); setName.invoke(o,"小猫"); System.out.println(o);
这段代码中,第三行的 `clazz.getMethod("setName",clazz)` 中,第二个参数应该是 `String.class`,而不是 `clazz`,因为 `setName` 方法的参数类型是 `String` 而不是 `Class`。
修改后的代码如下所示:
```
Constructor constructor = clazz.getConstructor();
Object o = constructor.newInstance();
Method setName = clazz.getMethod("setName", String.class);
setName.invoke(o, "小猫");
System.out.println(o);
```
这样就可以正确地调用 `setName` 方法并为对象设置名字了。
package step4; import java.lang.reflect.Constructor; import java.lang.reflect.InvocationTargetException; import java.lang.reflect.Method; public class Reflect_stu { public static void main(String[] args) throws InvocationTargetException { //使用反射调用 Class clazz = null; try { clazz = Class.forName("step4.Apple"); /********** Begin *********/ Method setPriceMethod = clazz.getMethod("setPrice", double.class); Constructor appleConstructor = clazz.getConstructor(); Object apple = appleConstructor.newInstance(); setPriceMethod.invoke(apple, 14); Method getPriceMethod = clazz.getMethod("getPrice"); System.out.println(getPriceMethod.invoke(apple)); Method getTotal = clazz.getMethod("getTotal", double.class, int.class); System.out.println(getTotal.invoke(apple, 20, 24)); /********** End *********/ } catch (Exception e) { e.printStackTrace(); } } } class Apple { private double price; private int count; public Apple() { } public double getPrice() { return price; } public void setPrice(double price) { this.price = price; } public int getCount() { return count; } public void setCount(int count) { this.count = count; } public double getTotal(double price, int count) { return price * count; } }
这段代码是一个使用反射调用的示例,它包括一个主类 Reflect_stu 和一个 Apple 类。在主类中,首先使用 Class.forName 方法获取 Apple 类的 Class 对象,然后使用 getMethod 方法获取 Apple 类中的 setPrice、getPrice 和 getTotal 方法,并使用 getConstructor 方法获取 Apple 类的默认构造方法的 Constructor 对象。接着使用 newInstance 方法创建一个 Apple 对象,并使用 invoke 方法调用 setPrice 方法设置 Apple 对象的价格为 14,使用 invoke 方法调用 getPrice 方法获取 Apple 对象的价格并输出,最后使用 invoke 方法调用 getTotal 方法计算 Apple 对象的总价并输出。
阅读全文
相关推荐
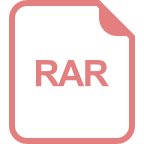
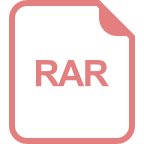

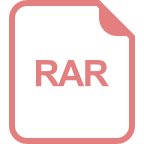
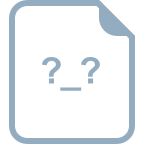
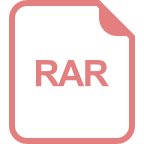
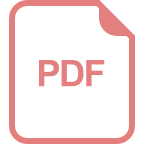



