写出redis分布式锁
时间: 2023-09-24 07:06:06 浏览: 40
Redis分布式锁的实现方法有很多种,以下是其中一种常见的实现方法:
1. 在Redis中创建一个key作为锁,例如:lockKey。
2. 使用SETNX命令尝试获取锁,如果返回结果为1,则表示获取锁成功,否则表示锁已被其他进程占用。
3. 设置锁的过期时间,防止进程崩溃或者死锁导致锁一直被占用。
4. 当进程需要释放锁时,使用DEL命令删除锁。
5. 如果获取锁失败,则需要等待一段时间后重新尝试获取锁,可以使用SLEEP命令来等待一段时间。
6. 如果获取锁的进程崩溃或者异常退出,可以使用Lua脚本来释放锁,避免死锁的发生。
以下是示例代码:
```python
import redis
import time
class RedisLock:
def __init__(self, redis_client, lock_key, timeout=10):
self.redis_client = redis_client
self.lock_key = lock_key
self.timeout = timeout
def acquire(self):
while True:
timestamp = int(time.time()) + self.timeout
if self.redis_client.setnx(self.lock_key, timestamp):
return True
current_timestamp = self.redis_client.get(self.lock_key)
if current_timestamp and int(current_timestamp) < int(time.time()):
old_timestamp = self.redis_client.getset(self.lock_key, timestamp)
if old_timestamp and old_timestamp == current_timestamp:
return True
time.sleep(0.1)
def release(self):
self.redis_client.delete(self.lock_key)
redis_client = redis.Redis(host='localhost', port=6379, db=0)
lock = RedisLock(redis_client, 'my_lock', 10)
if lock.acquire():
try:
# do some work here
pass
finally:
lock.release()
else:
# lock not acquired
pass
```
相关推荐
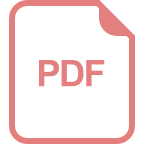
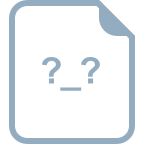
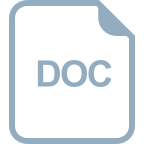
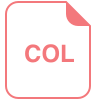
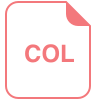
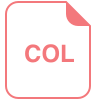
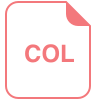
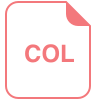









