.编写一个函数,接收一个字符串,统计其中任意两个及两个以上相邻字符相同的子串的个数。用c语言
时间: 2023-12-03 16:44:08 浏览: 35
可以使用暴力枚举的方法,从字符串的第一个字符开始,依次判断相邻两个字符是否相同,如果相同则继续向后判断,直到找到一个不同的字符为止,这时就找到了一个相同的子串。然后继续从下一个字符开始寻找。
具体实现如下:
```c
#include <stdio.h>
#include <string.h>
int count_substring(char *str) {
int len = strlen(str);
int count = 0;
for (int i = 0; i < len; i++) {
for (int j = i + 1; j < len; j++) {
if (str[j] != str[j-1]) {
break;
}
count++;
}
}
return count;
}
int main() {
char str[100];
printf("请输入一个字符串:");
scanf("%s", str);
int count = count_substring(str);
printf("相邻字符相同的子串个数为:%d\n", count);
return 0;
}
```
在上面的代码中,`count_substring` 函数接收一个字符串指针作为参数,返回相邻字符相同的子串个数。首先获取字符串的长度,然后使用两层循环遍历字符串,从第一个字符开始,依次判断相邻两个字符是否相同,如果相同则继续向后判断,直到找到一个不同的字符为止,这时就找到了一个相同的子串。子串个数加一,然后继续从下一个字符开始寻找。最后返回子串个数即可。
在主函数中,首先提示用户输入一个字符串,然后调用 `count_substring` 函数计算相邻字符相同的子串个数,并输出结果。
相关推荐
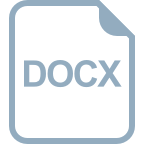
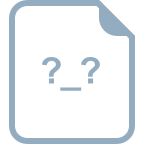
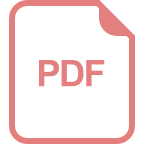








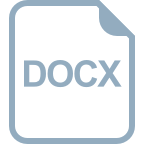
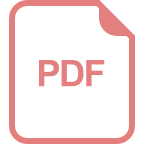
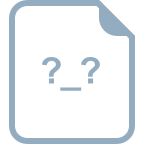
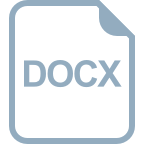
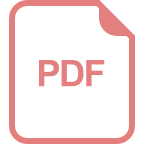
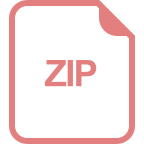