c语言结构体升序降序排列
时间: 2023-07-09 16:33:22 浏览: 55
要对结构体进行升序或者降序排序,可以使用标准库函数 `qsort`。下面是一个示例代码,包含升序和降序两种排序方式:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 定义一个结构体
struct student {
char name[20];
int age;
float score;
};
// 比较函数,用于 qsort 排序
int cmp_asc(const void *a, const void *b) {
struct student *s1 = (struct student *)a;
struct student *s2 = (struct student *)b;
if (s1->score < s2->score) {
return -1;
} else if (s1->score > s2->score) {
return 1;
} else {
return 0;
}
}
int cmp_desc(const void *a, const void *b) {
struct student *s1 = (struct student *)a;
struct student *s2 = (struct student *)b;
if (s1->score > s2->score) {
return -1;
} else if (s1->score < s2->score) {
return 1;
} else {
return 0;
}
}
int main() {
struct student stu[5] = {
{"Tom", 18, 89.5},
{"Jerry", 19, 78.5},
{"Bob", 20, 98.0},
{"Alice", 18, 75.0},
{"Lucy", 19, 88.0}
};
// 对结构体进行升序排序
qsort(stu, 5, sizeof(struct student), cmp_asc);
// 输出升序排序后的结果
printf("升序排序后的结果:\n");
for (int i = 0; i < 5; i++) {
printf("%s %d %.1f\n", stu[i].name, stu[i].age, stu[i].score);
}
// 对结构体进行降序排序
qsort(stu, 5, sizeof(struct student), cmp_desc);
// 输出降序排序后的结果
printf("降序排序后的结果:\n");
for (int i = 0; i < 5; i++) {
printf("%s %d %.1f\n", stu[i].name, stu[i].age, stu[i].score);
}
return 0;
}
```
上面的代码中,我们定义了一个 `struct student` 结构体,并且定义了两个比较函数 `cmp_asc` 和 `cmp_desc`,分别用于升序和降序排序。在 `main` 函数中,我们先使用 `qsort` 函数对结构体进行升序排序,然后输出升序排序后的结果;接着再使用 `qsort` 函数对结构体进行降序排序,最后输出降序排序后的结果。
相关推荐
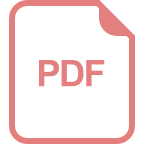










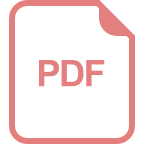