新建包,命名t3,按以下要求编写程序:设计一个学生类 Student 和它的一个子类 Undergraduate ,并进行测试,提示如下。 (1)创建学生类(命名: Student )属性: Name (姓名)和 Age (年龄);请将属性进行封装。定义两个构造方法:一个无参构造方法,一个包含两个参数的构造方法,用于给 Name 和 Age 属性赋值。定义一个 Show ()方法,完成打印 Student 的属性信息。 (2)创建本科生类(命名: Undergraduate )增加一个 Degree (学位)属性。 有一个包含三个参数的构造方法,前两个参数用于给继承的 Name 和 Age 属性赋值,第三个参数用于给 Degree 属性赋值,一个 Show ()方法打印 Undergraduate 的属性信息。 (3)创建测试类(命名 Test )分别创建 Student 对象和 Undergraduate 对象,并调用他们的 Show ()方法
时间: 2023-12-10 08:40:42 浏览: 38
可以按照以下方式实现:
1. 创建 Student 类:
```python
class Student:
def __init__(self):
self.__name = ""
self.__age = 0
def __init__(self, name, age):
self.__name = name
self.__age = age
def show(self):
print("Name:", self.__name)
print("Age:", self.__age)
```
2. 创建 Undergraduate 类,继承自 Student 类:
```python
class Undergraduate(Student):
def __init__(self, name, age, degree):
super().__init__(name, age)
self.__degree = degree
def show(self):
super().show()
print("Degree:", self.__degree)
```
3. 创建测试类 Test:
```python
class Test:
def run(self):
s = Student("Tom", 18)
s.show()
u = Undergraduate("Lucy", 20, "Bachelor")
u.show()
if __name__ == "__main__":
t = Test()
t.run()
```
运行测试类,输出结果为:
```
Name: Tom
Age: 18
Name: Lucy
Age: 20
Degree: Bachelor
```
相关推荐
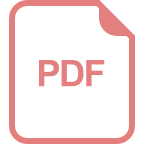
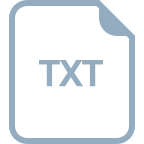
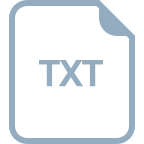















