inotify机制 文件创建检测
时间: 2023-07-19 17:07:50 浏览: 46
inotify是Linux内核提供的一种文件系统通知机制,可以用来监视文件系统的变化。其中包括文件的创建、删除、修改、移动等操作。使用inotify机制可以非常方便地实现对文件系统变化的监控,比如实时同步文件夹、监控日志文件等应用场景。
对于文件的创建检测,我们可以使用inotify机制中的IN_CREATE事件来实现。当一个新文件被创建时,内核会向监听该目录的进程发送一个IN_CREATE事件通知。进程可以通过inotify API来获取该事件通知,并进行相应的处理。
以下是一个使用inotify机制进行文件创建监控的示例代码:
```
#include <stdio.h>
#include <stdlib.h>
#include <errno.h>
#include <sys/types.h>
#include <sys/inotify.h>
#define EVENT_SIZE (sizeof(struct inotify_event))
#define BUF_LEN (1024 * (EVENT_SIZE + 16))
int main(int argc, char **argv)
{
int fd, wd;
char buf[BUF_LEN];
ssize_t len;
struct inotify_event *event;
if(argc < 2){
fprintf(stderr, "Usage: %s <dir>\n", argv[0]);
exit(EXIT_FAILURE);
}
fd = inotify_init();
if(fd == -1){
perror("inotify_init");
exit(EXIT_FAILURE);
}
wd = inotify_add_watch(fd, argv[1], IN_CREATE);
if(wd == -1){
perror("inotify_add_watch");
exit(EXIT_FAILURE);
}
printf("Watching '%s' for new files...\n", argv[1]);
while(1){
len = read(fd, buf, BUF_LEN);
if(len == -1 && errno != EAGAIN){
perror("read");
exit(EXIT_FAILURE);
}
if(len <= 0)
continue;
event = (struct inotify_event *)buf;
while(len > 0){
if(event->mask & IN_CREATE){
printf("New file '%s' created.\n", event->name);
}
len -= EVENT_SIZE + event->len;
event = (struct inotify_event *)((char *)event + EVENT_SIZE + event->len);
}
}
exit(EXIT_SUCCESS);
}
```
运行该程序时,需要指定要监控的目录作为命令行参数,例如:
```
./inotify_test /tmp
```
该程序会不断地监控指定目录下的文件创建事件,并在控制台输出新文件的名称。
相关推荐
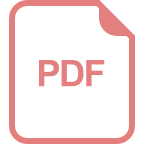
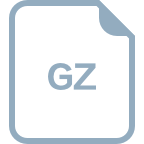









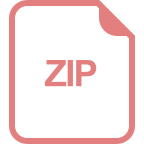
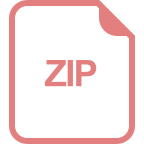
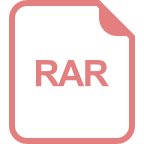
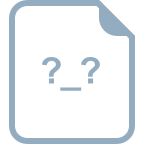