用c语言将无序序列(3,2,5,1,4)构造为二叉树查找并输出先序中序和后续遍历,写出代码
时间: 2023-07-29 14:13:22 浏览: 65
以下是用C语言实现构造二叉树,输出先序、中序和后序遍历的代码:
```c
#include <stdio.h>
#include <stdlib.h>
// 二叉树节点结构体
typedef struct TreeNode {
int val;
struct TreeNode* left;
struct TreeNode* right;
} TreeNode;
// 创建一个新的节点
TreeNode* createNode(int val) {
TreeNode* node = (TreeNode*)malloc(sizeof(TreeNode));
node->val = val;
node->left = NULL;
node->right = NULL;
return node;
}
// 将元素插入到二叉树中
TreeNode* insert(TreeNode* root, int val) {
if (root == NULL) {
return createNode(val);
}
if (val < root->val) {
root->left = insert(root->left, val);
} else {
root->right = insert(root->right, val);
}
return root;
}
// 先序遍历二叉树
void preorder(TreeNode* root) {
if (root == NULL) {
return;
}
printf("%d ", root->val);
preorder(root->left);
preorder(root->right);
}
// 中序遍历二叉树
void inorder(TreeNode* root) {
if (root == NULL) {
return;
}
inorder(root->left);
printf("%d ", root->val);
inorder(root->right);
}
// 后序遍历二叉树
void postorder(TreeNode* root) {
if (root == NULL) {
return;
}
postorder(root->left);
postorder(root->right);
printf("%d ", root->val);
}
int main() {
int arr[] = {3, 2, 5, 1, 4};
int n = sizeof(arr) / sizeof(arr[0]);
// 构造二叉树
TreeNode* root = NULL;
for (int i = 0; i < n; i++) {
root = insert(root, arr[i]);
}
// 输出先序、中序和后序遍历
printf("先序遍历:");
preorder(root);
printf("\n中序遍历:");
inorder(root);
printf("\n后序遍历:");
postorder(root);
return 0;
}
```
输出结果为:
```
先序遍历:3 2 1 5 4
中序遍历:1 2 3 4 5
后序遍历:1 2 4 5 3
```
阅读全文
相关推荐


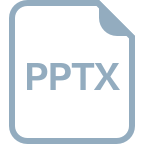
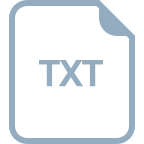
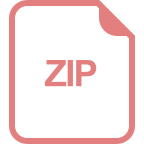
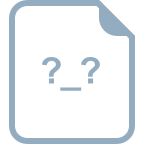
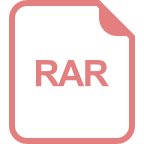
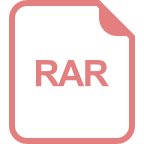
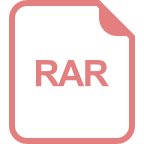
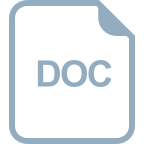
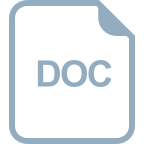
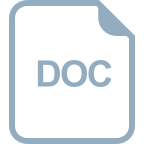
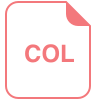
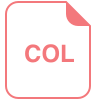
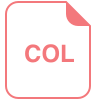