python使用区域生长法分割图像
时间: 2023-09-24 21:14:08 浏览: 105
区域生长法是一种基于像素相似性的图像分割方法,其基本思想是从一个或多个种子点开始,通过递归地合并相邻像素,直到所有像素分成若干个不同的区域为止。Python中可以使用OpenCV库实现区域生长法图像分割。
下面是一个使用OpenCV实现区域生长法图像分割的示例代码:
```python
import cv2
# 读取图像
img = cv2.imread('image.jpg')
# 预处理图像
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
blur = cv2.GaussianBlur(gray, (5, 5), 0)
# 区域生长法分割图像
seed_points = [(100, 100)]
connected_pixels = []
for seed in seed_points:
# 扩展种子点
neighbors = [(seed[0] - 1, seed[1] - 1),
(seed[0] - 1, seed[1]),
(seed[0] - 1, seed[1] + 1),
(seed[0], seed[1] - 1),
(seed[0], seed[1] + 1),
(seed[0] + 1, seed[1] - 1),
(seed[0] + 1, seed[1]),
(seed[0] + 1, seed[1] + 1)]
for neighbor in neighbors:
if 0 <= neighbor[0] < img.shape[0] and 0 <= neighbor[1] < img.shape[1]:
if neighbor not in seed_points and neighbor not in connected_pixels:
# 判断相邻像素是否属于同一区域
if abs(int(blur[seed]) - int(blur[neighbor])) < 50:
connected_pixels.append(neighbor)
seed_points.append(neighbor)
# 绘制分割结果
for pixel in connected_pixels:
img[pixel] = (0, 255, 0)
# 显示分割结果
cv2.imshow('Segmentation Result', img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
在上述代码中,我们首先读取图像,并对其进行预处理,包括转换为灰度图像和高斯模糊。接着,我们选择一个种子点作为起点,递归地寻找与之相邻的像素,并通过像素相似性判断是否属于同一区域。最终,我们将分割结果绘制到原图像上,并显示出来。
需要注意的是,区域生长法对图像的预处理和参数设置非常敏感,可能需要根据具体情况进行调整。此外,该方法也存在一定的局限性,例如无法处理非连通区域等情况。
阅读全文
相关推荐




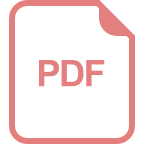












