winform中导出多个数据到excel表格并关闭,如何提高执行效率
时间: 2024-02-25 15:52:11 浏览: 89
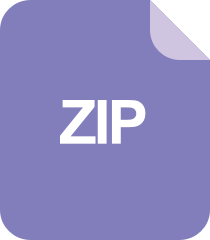
winform导出数据到excel
在 Winform 中导出多个数据到 Excel 表格并关闭,可以采用多线程的方式来提高执行效率。具体操作步骤如下:
1. 创建一个线程池,用于执行导出操作。
```csharp
int threadCount = Environment.ProcessorCount; //获取 CPU 核心数
ThreadPool.SetMaxThreads(threadCount, threadCount); //设置线程池最大线程数
```
2. 遍历数据源,为每个数据源创建一个线程,并将其加入到线程池中。
```csharp
foreach (var dataSource in dataSources)
{
ThreadPool.QueueUserWorkItem(state =>
{
ExportToExcel(dataSource, filePath);
Interlocked.Decrement(ref runningThreadCount); //线程执行完毕,运行线程数减1
});
Interlocked.Increment(ref runningThreadCount); //新建线程,运行线程数加1
}
```
3. 在 UI 线程中,等待所有导出操作执行完毕,并关闭 Excel 进程。
```csharp
while (runningThreadCount > 0)
{
Application.DoEvents(); //让主线程处理消息
}
excelApp.Quit(); //关闭 Excel 进程
```
完整的代码示例如下:
```csharp
using System;
using System.Collections.Generic;
using System.IO;
using System.Threading;
using Microsoft.Office.Interop.Excel;
using NPOI.HSSF.UserModel;
//定义数据源
public class Person
{
public string Name { get; set; }
public int Age { get; set; }
public string Gender { get; set; }
}
public static void ExportToExcel(Person[] dataSource, string filePath)
{
//创建一个 Excel 文件并设置其属性
HSSFWorkbook workbook = new HSSFWorkbook();
HSSFSheet sheet = workbook.CreateSheet("Sheet1");
//创建表头信息
HSSFRow headerRow = sheet.CreateRow(0);
headerRow.CreateCell(0).SetCellValue("姓名");
headerRow.CreateCell(1).SetCellValue("年龄");
headerRow.CreateCell(2).SetCellValue("性别");
//遍历数据源,将数据写入到 Excel 文件中
for (int i = 0; i < dataSource.Length; i++)
{
HSSFRow dataRow = sheet.CreateRow(i + 1);
dataRow.CreateCell(0).SetCellValue(dataSource[i].Name);
dataRow.CreateCell(1).SetCellValue(dataSource[i].Age);
dataRow.CreateCell(2).SetCellValue(dataSource[i].Gender);
}
//将 Excel 文件保存到本地
using (FileStream fileStream = new FileStream(filePath, FileMode.Create))
{
workbook.Write(fileStream);
}
}
public static void ExportToExcel(List<Person[]> dataSources, string folderPath)
{
//创建 Excel 应用程序对象
Application excelApp = new Application();
excelApp.DisplayAlerts = false; //禁止 Excel 弹出警告框
int threadCount = Environment.ProcessorCount; //获取 CPU 核心数
ThreadPool.SetMaxThreads(threadCount, threadCount); //设置线程池最大线程数
int runningThreadCount = 0; //记录正在运行的线程数
//遍历数据源,为每个数据源创建一个线程,并将其加入到线程池中
foreach (var dataSource in dataSources)
{
string fileName = Guid.NewGuid().ToString() + ".xls"; //生成一个随机文件名
string filePath = Path.Combine(folderPath, fileName); //生成文件的完整路径
ThreadPool.QueueUserWorkItem(state =>
{
ExportToExcel(dataSource, filePath);
Interlocked.Decrement(ref runningThreadCount); //线程执行完毕,运行线程数减1
});
Interlocked.Increment(ref runningThreadCount); //新建线程,运行线程数加1
}
//在 UI 线程中,等待所有导出操作执行完毕,并关闭 Excel 进程
while (runningThreadCount > 0)
{
Application.DoEvents(); //让主线程处理消息
}
excelApp.Quit(); //关闭 Excel 进程
}
```
通过多线程的方式,可以同时处理多个数据源的导出操作,从而提高执行效率。需要注意的是,在使用多线程的情况下,我们需要在 UI 线程中等待所有操作执行完毕,再关闭 Excel 进程。
阅读全文
相关推荐
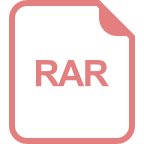
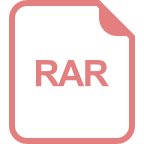
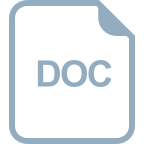
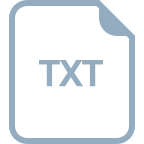
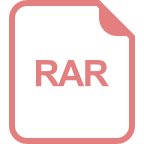
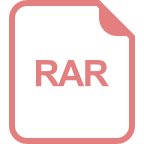
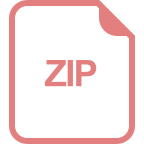
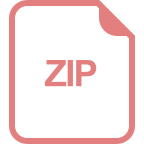
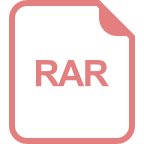
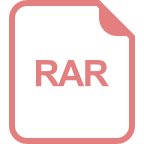
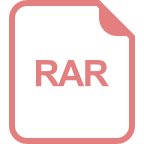
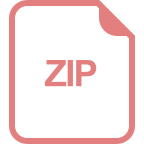
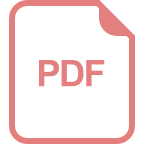
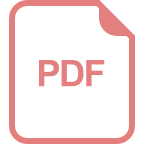
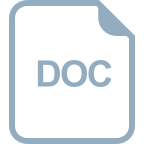
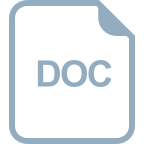