编写字符串运算符“+=”的重载函数,使运算符“+=”用于字符串的拼接运算,实现字符串的S1+=S2操作运算。分别用友元函数和成员函数实现。
时间: 2024-03-22 11:38:57 浏览: 62
友元函数实现:
```cpp
#include <iostream>
#include <cstring>
using namespace std;
class String {
private:
char *str;
public:
String(const char *s = NULL) {
if (s == NULL) {
str = new char[1];
str[0] = '\0';
} else {
str = new char[strlen(s) + 1];
strcpy(str, s);
}
}
~String() {
delete[] str;
}
void display() const {
cout << str << endl;
}
friend String operator+=(String &s1, const String &s2); // 友元函数
};
String operator+=(String &s1, const String &s2) {
char *temp = new char[strlen(s1.str) + strlen(s2.str) + 1];
strcpy(temp, s1.str);
strcat(temp, s2.str);
delete[] s1.str;
s1.str = temp;
return s1;
}
int main() {
String s1("Hello, ");
String s2("world!");
s1 += s2;
s1.display();
return 0;
}
```
成员函数实现:
```cpp
#include <iostream>
#include <cstring>
using namespace std;
class String {
private:
char *str;
public:
String(const char *s = NULL) {
if (s == NULL) {
str = new char[1];
str[0] = '\0';
} else {
str = new char[strlen(s) + 1];
strcpy(str, s);
}
}
~String() {
delete[] str;
}
void display() const {
cout << str << endl;
}
String &operator+=(const String &s) { // 成员函数
char *temp = new char[strlen(str) + strlen(s.str) + 1];
strcpy(temp, str);
strcat(temp, s.str);
delete[] str;
str = temp;
return *this;
}
};
int main() {
String s1("Hello, ");
String s2("world!");
s1 += s2;
s1.display();
return 0;
}
```
阅读全文
相关推荐
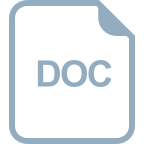
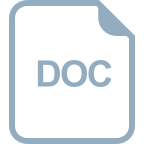
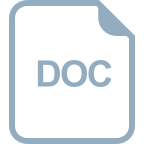

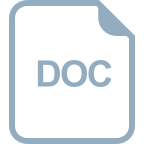
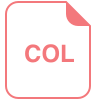
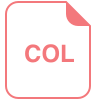
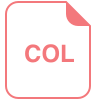


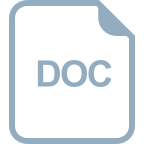
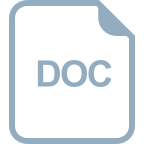
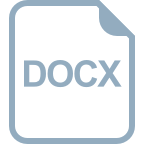
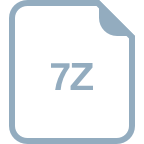
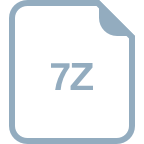
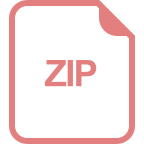