c++多线程或异步编程技术代码示例
时间: 2024-01-29 17:03:16 浏览: 90
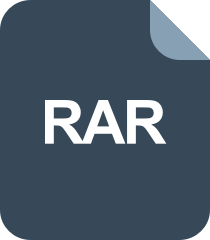
chuankou.rar_进程与线程_Visual_C++_
下面是使用C++语言实现多线程和异步编程的代码示例:
多线程示例:
```cpp
#include <iostream>
#include <thread>
// 线程函数,打印数字
void printNumbers() {
for (int i = 1; i <= 10; ++i) {
std::cout << i << std::endl;
}
}
// 线程函数,打印字母
void printLetters() {
for (char letter : {'a', 'b', 'c', 'd', 'e'}) {
std::cout << letter << std::endl;
}
}
int main() {
// 创建两个线程
std::thread thread1(printNumbers);
std::thread thread2(printLetters);
// 等待两个线程执行完毕
thread1.join();
thread2.join();
std::cout << "Done!" << std::endl;
return 0;
}
```
上述代码创建了两个线程,一个打印数字,一个打印字母。两个线程可以并行执行,提高了程序的性能。
异步编程示例:
```cpp
#include <iostream>
#include <string>
#include <future>
// 异步函数,获取网站HTML内容
std::string fetchWebsite(const std::string& url) {
// 省略异步获取HTML内容的代码
return "HTML content";
}
int main() {
std::string url = "http://www.example.com";
// 异步获取网站HTML内容
std::future<std::string> futureHTML = std::async(std::launch::async, fetchWebsite, url);
// 程序可以继续执行其他任务,不必等待HTML内容返回
std::cout << "Program continues..." << std::endl;
// 等待HTML内容返回,并打印出来
std::string html = futureHTML.get();
std::cout << html << std::endl;
return 0;
}
```
上述代码使用了C++11标准的异步编程特性,异步获取一个网站的HTML内容,并打印出来。异步编程可以提高程序的响应速度和并发性。
阅读全文
相关推荐
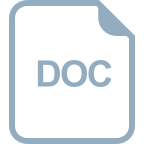
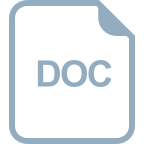
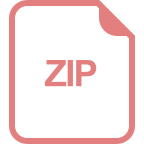
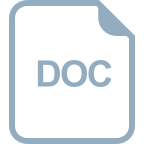
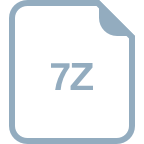
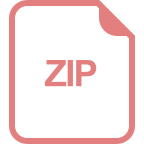
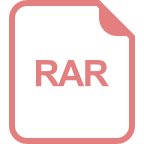
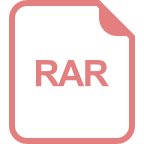
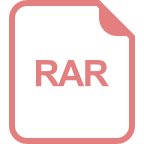
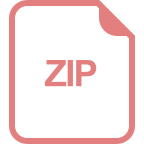
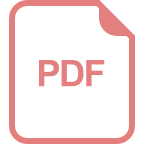
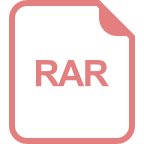
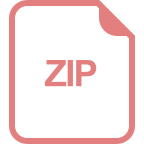
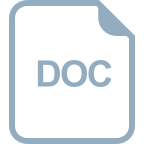
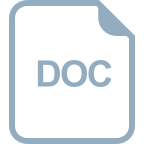
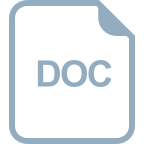
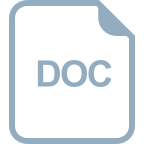
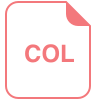
