以下代码存在不可以自动反向传播的bug,为什么: # 前向LSTM outputs_forward = [] for t in range(input.size(0)): x = input[t] i = torch.sigmoid(torch.matmul(x, self.W_i_forward) + torch.matmul(h_forward[-1], self.U_i_forward) + self.b_i_forward) f = torch.sigmoid(torch.matmul(x, self.W_f_forward) + torch.matmul(h_forward[-1], self.U_f_forward) + self.b_f_forward) c_tilde = torch.tanh(torch.matmul(x, self.W_c_forward) + torch.matmul(h_forward[-1], self.U_c_forward) + self.b_c_forward) c_forward[-1] = f * c_forward[-1] + i * c_tilde o = torch.matmul(x, self.W_o_forward) + torch.matmul(h_forward[-1], self.U_o_forward) + self.b_o_forward o = torch.sigmoid(o) h_forward[-1] = o * torch.tanh(c_forward[-1]) outputs_forward.append(h_forward[-1])
时间: 2024-03-03 12:49:45 浏览: 140
这段代码存在一个不能自动反向传播的bug,原因是在循环中对h_forward和c_forward的值进行了修改。在PyTorch中,只有被标记为requires_grad=True的张量才能够自动求梯度,而对张量进行in-place操作会导致梯度信息丢失,从而无法进行自动求梯度。
具体来说,在这段代码中,对h_forward和c_forward的修改会导致后续的梯度计算出现问题,从而无法进行自动反向传播。解决这个问题的方法是创建一个新的变量来保存h_forward和c_forward的值,而不是直接修改它们的值。修改后的代码如下:
```python
# 前向LSTM
outputs_forward = []
h_forward_new = h_forward.clone()
c_forward_new = c_forward.clone()
for t in range(input.size(0)):
x = input[t]
i = torch.sigmoid(torch.matmul(x, self.W_i_forward) + torch.matmul(h_forward_new[-1], self.U_i_forward) + self.b_i_forward)
f = torch.sigmoid(torch.matmul(x, self.W_f_forward) + torch.matmul(h_forward_new[-1], self.U_f_forward) + self.b_f_forward)
c_tilde = torch.tanh(torch.matmul(x, self.W_c_forward) + torch.matmul(h_forward_new[-1], self.U_c_forward) + self.b_c_forward)
c_forward_new[-1] = f * c_forward_new[-1] + i * c_tilde
o = torch.matmul(x, self.W_o_forward) + torch.matmul(h_forward_new[-1], self.U_o_forward) + self.b_o_forward
o = torch.sigmoid(o)
h_forward_new[-1] = o * torch.tanh(c_forward_new[-1])
outputs_forward.append(h_forward_new[-1])
h_forward_new = torch.cat([h_forward_new[1:], h_forward_new[-1].unsqueeze(0)])
c_forward_new = torch.cat([c_forward_new[1:], c_forward_new[-1].unsqueeze(0)])
h_forward = h_forward_new
c_forward = c_forward_new
```
在修改后的代码中,我们先创建了新的变量h_forward_new和c_forward_new来保存h_forward和c_forward的值,并使用clone()方法复制张量的值。然后,在循环中,我们使用h_forward_new和c_forward_new来进行前向计算,并将计算得到的输出保存到outputs_forward中。最后,我们使用torch.cat()方法来更新h_forward_new和c_forward_new的值,并将其赋值给h_forward和c_forward。这样就可以避免in-place操作导致的梯度丢失问题。
阅读全文
相关推荐
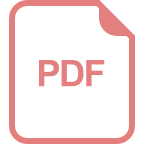
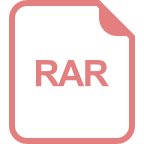
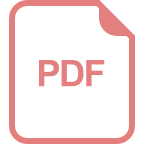





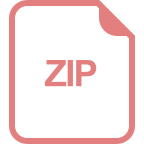
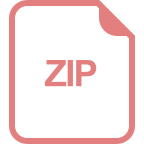
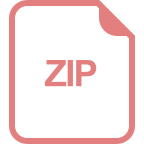
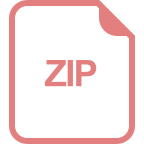
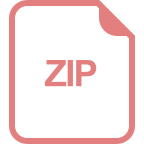
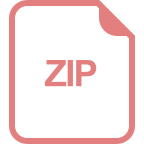
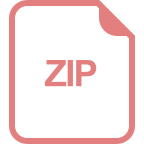
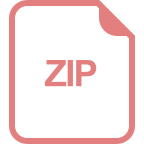
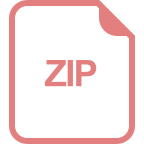
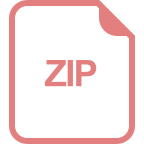
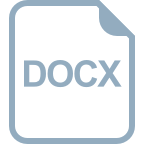