react 使用 js 调用 link 点击
时间: 2024-01-13 07:04:52 浏览: 102
如果你想要使用 JavaScript 来模拟 link 的点击事件,可以使用 `click()` 方法来实现。具体实现如下:
```javascript
const link = document.querySelector('a'); // 获取 link 元素
link.click(); // 模拟 link 点击事件
```
如果你想在 React 中使用这个方法,可以在组件的 `render` 方法中使用 `ref` 来获取 link 元素,并在适当的时候调用 `click()` 方法。具体实现如下:
```javascript
class MyComponent extends React.Component {
handleClick = () => {
this.link.click(); // 在点击事件中调用 click() 方法
}
render() {
return (
<div>
<a href="https://www.example.com" ref={link => this.link = link}>Link</a>
<button onClick={this.handleClick}>Click me</button>
</div>
);
}
}
```
在上面的代码中,我们使用 `ref` 来获取 link 元素,并将其保存在组件实例的属性中。然后在按钮的点击事件中调用 `click()` 方法来模拟 link 的点击事件。
相关问题
react-router 2.8.1 js 点击事件 跳转
要实现路由跳转,你需要先安装 react-router-dom 模块。
然后在你的组件中,可以使用 Link 组件来实现路由跳转。例如:
```jsx
import React from 'react';
import { Link } from 'react-router-dom';
function MyComponent() {
return (
<div>
<h1>Hello World!</h1>
<Link to="/other-page">Go to other page</Link>
</div>
);
}
```
在上面的代码中,当用户点击 "Go to other page" 链接时,会自动跳转到 "/other-page" 路由。你需要在你的路由配置中定义 "/other-page" 路由对应的组件。
如果你需要在 JS 中编程式地实现路由跳转,可以使用 history 对象。例如:
```jsx
import React from 'react';
import { useHistory } from 'react-router-dom';
function MyComponent() {
const history = useHistory();
function handleClick() {
history.push('/other-page');
}
return (
<div>
<h1>Hello World!</h1>
<button onClick={handleClick}>Go to other page</button>
</div>
);
}
```
在上面的代码中,当用户点击 "Go to other page" 按钮时,会调用 handleClick 函数,该函数会使用 history.push 方法来实现路由跳转。
阅读全文
相关推荐
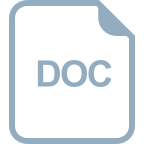
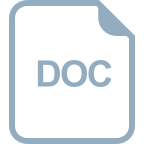
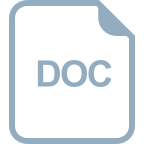
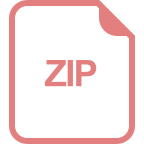
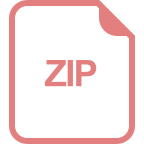
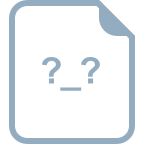
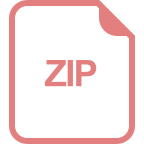
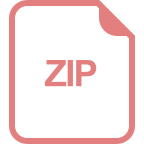
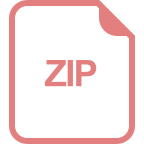
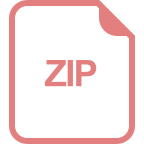
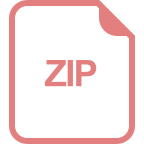
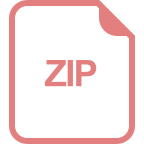
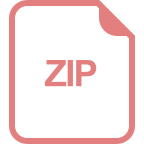
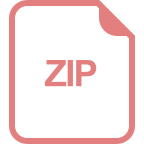
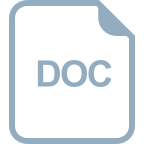
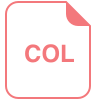
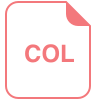
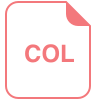