优化以下方法: private Token nextToken() { Token result; if (!hasEnd) { if (index < source.length()) { switch (source.charAt(index)) { case '.': result = new Token(TokenType.DOT, source.charAt(index)); // System.out.println("DOT"); break; case '\': result = new Token(TokenType.LAMBDA, source.charAt(index)); // System.out.println("LAMBDA"); break; case '(': result = new Token(TokenType.LPAREN, source.charAt(index)); // System.out.println("LPAREN"); break; case ')': result = new Token(TokenType.RPAREN, source.charAt(index)); // System.out.println("RPAREN"); break; case ' ': result = new Token(TokenType.BLANK, source.charAt(index)); break; default: if ('a' <= source.charAt(index) && source.charAt(index) <= 'z') { int temp = index + 1; while (temp < source.length() && (('a' <= source.charAt(temp) && source.charAt(temp) <= 'z') || ('A' <= source.charAt(temp) && source.charAt(temp) <= 'Z'|| ('0' <= source.charAt(temp) && source.charAt(temp) <= '9')))) temp++; result = new Token(TokenType.LCID, source.substring(index, temp)); // System.out.println("LCID"); } else { result = new Token(TokenType.EOF, ""); // System.out.println("EOF"); } } } else { result = new Token(TokenType.EOF, ""); System.out.println("EOF"); hasEnd = true; } } else result = new Token(TokenType.EOF, ""); return result; }
时间: 2024-01-05 10:03:02 浏览: 111
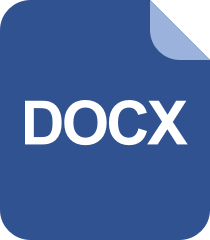
优化的问题
可以考虑以下优化:
1. 减少重复代码
可以将 `result = new Token(TokenType.xxx, source.charAt(index));` 的代码抽取出来,减少重复的代码。
2. 减少字符串拼接
在处理 `LCID` 类型的 token 时,使用了 `source.substring(index, temp)` 将子串拼接成新的字符串。这个操作比较耗时,可以考虑将 `LCID` 类型 token 的值存储为起始和结束索引,而不是字符串。
3. 使用 Map 存储字符类型
将每个字符对应的 token 类型存储到一个 Map 中,这样可以避免使用多个 if/else 语句判断每个字符的类型。
综上所述,可以将代码修改为以下形式:
```
private static final Map<Character, TokenType> TOKEN_MAP = new HashMap<>();
static {
TOKEN_MAP.put('.', TokenType.DOT);
TOKEN_MAP.put('\\', TokenType.LAMBDA);
TOKEN_MAP.put('(', TokenType.LPAREN);
TOKEN_MAP.put(')', TokenType.RPAREN);
TOKEN_MAP.put(' ', TokenType.BLANK);
}
private Token nextToken() {
Token result;
if (!hasEnd) {
if (index < source.length()) {
char ch = source.charAt(index);
TokenType type = TOKEN_MAP.get(ch);
if (type != null) {
result = new Token(type, ch);
} else if ('a' <= ch && ch <= 'z') {
int end = index + 1;
while (end < source.length()) {
char nextCh = source.charAt(end);
if (!('a' <= nextCh && nextCh <= 'z') &&
!('A' <= nextCh && nextCh <= 'Z') &&
!('0' <= nextCh && nextCh <= '9')) {
break;
}
end++;
}
result = new Token(TokenType.LCID, index, end - 1);
} else {
result = new Token(TokenType.EOF, "");
}
} else {
result = new Token(TokenType.EOF, "");
System.out.println("EOF");
hasEnd = true;
}
} else {
result = new Token(TokenType.EOF, "");
}
return result;
}
```
其中 `Token` 类的定义可以修改为:
```
class Token {
enum TokenType {
DOT, LAMBDA, LPAREN, RPAREN, BLANK, LCID, EOF
}
final TokenType type;
final char value;
final int start; // LCID 类型 token 的起始索引
final int end; // LCID 类型 token 的结束索引
Token(TokenType type, char value) {
this(type, value, -1, -1);
}
Token(TokenType type, int start, int end) {
this(type, '\0', start, end);
}
Token(TokenType type, char value, int start, int end) {
this.type = type;
this.value = value;
this.start = start;
this.end = end;
}
}
```
阅读全文
相关推荐
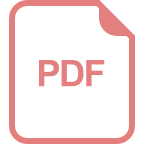
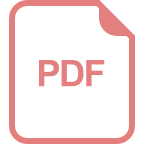

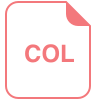
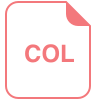
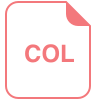
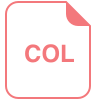
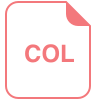
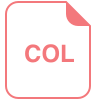
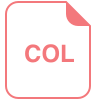
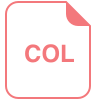
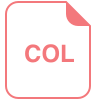
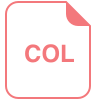
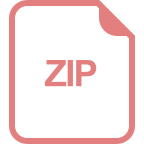
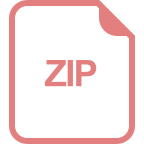