python遗传优化算法
时间: 2023-09-30 08:08:36 浏览: 54
遗传优化算法是一种基于生物进化过程的优化算法,通过模拟生物进化过程中的遗传、交叉、变异等操作,优化目标函数,以求解最优化问题。
在 Python 中,可以使用遗传优化算法库 DEAP 来实现遗传优化算法。DEAP 提供了丰富的遗传算法工具和算子,可以方便地进行遗传算法的构建和求解。
以下是使用 DEAP 实现遗传优化算法的基本步骤:
1. 定义问题:定义目标函数、变量范围等问题参数。
2. 定义个体编码方式:可以使用二进制、实数等编码方式来表示个体。
3. 定义适应度函数:根据问题的目标函数,定义适应度函数来评价个体的适应性。
4. 定义遗传算子:包括选择算子、交叉算子、变异算子等。
5. 初始化种群:随机生成一定数量的个体,作为初始种群。
6. 迭代演化:通过遗传算子对种群进行交叉、变异等操作,生成新一代种群,并根据适应度函数对新种群进行评价。
7. 判断终止条件:当满足一定的终止条件时,停止迭代,输出最优解。
下面是一个简单的 Python 代码示例:
```python
import random
from deap import base, creator, tools
# 定义问题
def fitness_func(individual):
return sum(individual), # 返回一个元组,因为 DEAP 要求适应度函数返回一个可迭代对象
toolbox = base.Toolbox()
# 定义个体编码方式
toolbox.register("attr_bool", random.randint, 0, 1)
toolbox.register("individual", tools.initRepeat, creator.Individual, toolbox.attr_bool, n=10)
# 定义适应度函数
creator.create("FitnessMax", base.Fitness, weights=(1.0,))
toolbox.register("evaluate", fitness_func)
# 定义遗传算子
toolbox.register("mate", tools.cxTwoPoint)
toolbox.register("mutate", tools.mutFlipBit, indpb=0.05)
toolbox.register("select", tools.selTournament, tournsize=3)
# 初始化种群
pop = toolbox.population(n=50)
# 迭代演化
for g in range(100):
# 评价种群
fitnesses = map(toolbox.evaluate, pop)
for ind, fit in zip(pop, fitnesses):
ind.fitness.values = fit
# 选择下一代种群
offspring = toolbox.select(pop, len(pop))
# 复制下一代种群
offspring = list(map(toolbox.clone, offspring))
# 对下一代种群进行交叉和变异
for child1, child2 in zip(offspring[::2], offspring[1::2]):
if random.random() < 0.5:
toolbox.mate(child1, child2)
del child1.fitness.values
del child2.fitness.values
for mutant in offspring:
if random.random() < 0.05:
toolbox.mutate(mutant)
del mutant.fitness.values
# 将新一代种群替换原来的种群
pop[:] = offspring
# 输出最优解
best_ind = tools.selBest(pop, 1)[0]
print("Best individual is: %s\nFitness value: %s" % (best_ind, best_ind.fitness.values[0]))
```
这个示例程序实现的是一个简单的二进制遗传算法,求解的是最大化一串二进制数中 1 的个数的问题。可以根据实际问题进行相应的修改和调整。
相关推荐
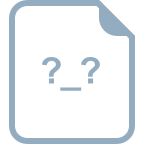














